Python is a popular programming language for web applications, data science, and machine learning due to its simplicity and readability. However, its ease of use does not automatically ensure security. By following secure coding practices, developers can write code that minimizes vulnerabilities and resists potential attacks.
In this guide, we’ll discuss fundamental principles for secure coding in Python, covering best practices for handling data, authentication, and common vulnerabilities. By integrating these practices into your development workflow, you can build applications that are not only functional but also secure.
Table of Contents
- Why Secure Coding Matters
- Input Validation and Sanitization
- Handling Sensitive Data Securely
- Preventing Common Vulnerabilities
- SQL Injection
- Cross-Site Scripting (XSS)
- Cross-Site Request Forgery (CSRF)
- Insecure Deserialization
- Best Practices for Authentication and Authorization
- Using Secure Libraries and Frameworks
- Error Handling and Logging
- Regular Security Audits and Code Reviews
- Conclusion
1. Why Secure Coding Matters
Security breaches can lead to severe consequences, including data theft, financial loss, and reputational damage. Secure coding practices aim to reduce the attack surface of an application by proactively addressing potential vulnerabilities. For Python developers, following secure coding standards is essential, as Python’s dynamic nature can sometimes mask subtle issues that lead to security weaknesses.
2. Input Validation and Sanitization
Validating User Input
Input validation ensures that the data provided by users meets the expected format, preventing injection attacks, buffer overflows, and other exploits. It’s essential to validate inputs on the server side, as client-side validation alone can be easily bypassed by attackers.
For example, if you’re expecting an integer input from a user, validate it with a try-except block to handle unexpected data types:
def validate_age(age_input): try: age = int(age_input) if age < 0: raise ValueError("Age cannot be negative.") return age except ValueError: raise Exception("Invalid input; please enter a valid number.")
Avoiding Injection Attacks
Sanitize user inputs by using parameterized queries when interacting with databases. This prevents SQL injection attacks, which occur when an attacker inserts malicious SQL code into an input field.
import sqlite3 def get_user(username): connection = sqlite3.connect("users.db") cursor = connection.cursor() # Use parameterized queries to prevent SQL injection cursor.execute("SELECT * FROM users WHERE username = ?", (username,)) user = cursor.fetchone() connection.close() return user
3. Handling Sensitive Data Securely
Using Environment Variables for Sensitive Information
Sensitive information such as API keys, database passwords, and encryption keys should never be hard-coded in source code. Use environment variables to store sensitive data and access them with os.environ
.
import os database_password = os.getenv("DATABASE_PASSWORD")
Tools like dotenv in Python can help manage environment variables, especially in development and deployment environments.
Encrypting Data
Use encryption libraries like cryptography or PyCrypto for securely encrypting sensitive data. For example, to store passwords, always use hashed values rather than plain text, employing algorithms like bcrypt or argon2.
from bcrypt import hashpw, gensalt password = "user_password".encode('utf-8') hashed = hashpw(password, gensalt())
4. Preventing Common Vulnerabilities
SQL Injection
SQL injection is a common vulnerability that occurs when untrusted input is directly used in SQL statements. Using parameterized queries is the best way to prevent this.
Cross-Site Scripting (XSS)
XSS attacks happen when an attacker injects malicious JavaScript into a web application. To prevent XSS, always sanitize user input and escape output that is rendered on web pages. For web frameworks like Flask or Django, use templating systems that automatically escape HTML by default.
Cross-Site Request Forgery (CSRF)
CSRF attacks trick users into submitting unauthorized requests. To prevent CSRF, use CSRF tokens for forms and verify them on the server side. Web frameworks like Django have built-in CSRF protection features.
Insecure Deserialization
Insecure deserialization happens when untrusted data is deserialized, allowing attackers to execute arbitrary code. Avoid using pickle
with untrusted data. Instead, use safer alternatives like JSON for data serialization.
import json data = {"name": "Alice", "age": 25} serialized_data = json.dumps(data) deserialized_data = json.loads(serialized_data)
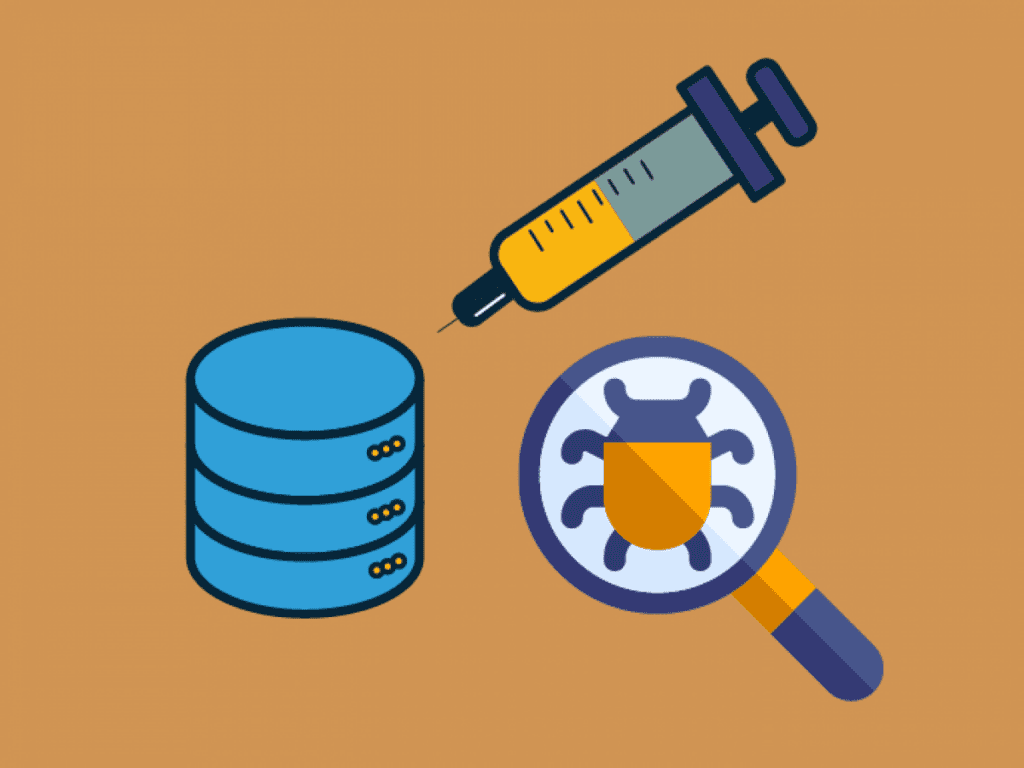
5. Best Practices for Authentication and Authorization
Authentication and authorization are core components of application security. Here are some best practices:
- Use Secure Password Storage: Store passwords using salted hashes and avoid plain-text storage. Libraries like bcrypt and argon2 provide secure hashing algorithms.
- Implement Multi-Factor Authentication (MFA): Adding MFA is an extra layer of security that reduces the risk of unauthorized access.
- Limit Login Attempts: To protect against brute-force attacks, limit the number of login attempts and implement account lockout after a set number of failures.
- Implement Role-Based Access Control (RBAC): For complex applications, use RBAC to enforce fine-grained access control.
6. Using Secure Libraries and Frameworks
Libraries and frameworks can reduce development time but can also introduce vulnerabilities if not maintained. Follow these best practices when selecting third-party libraries:
- Verify Library Security: Check for known vulnerabilities in third-party libraries using tools like Dependabot or Snyk.
- Update Regularly: Regularly update dependencies to the latest versions, which often include security patches.
- Limit Access: Use only the permissions and features you need to minimize the attack surface.
7. Error Handling and Logging
Logging
Logging can reveal sensitive information if not handled correctly. Avoid logging sensitive data such as passwords, credit card numbers, or personally identifiable information (PII). Ensure logs are stored securely, and access is restricted.
import logging logging.basicConfig(filename='app.log', level=logging.INFO) logging.info("User accessed the application") # Avoid sensitive data here
Error Handling
Do not expose detailed error messages to end users, as they may reveal implementation details. Use custom error messages to handle exceptions gracefully while maintaining security.
try: # Your code here except Exception as e: logging.error(f"An error occurred: {str(e)}") print("An unexpected error occurred. Please try again later.")
8. Regular Security Audits and Code Reviews
Code reviews and security audits help identify vulnerabilities and improve code quality. Implementing these practices can prevent security risks before they reach production.
Tools for Security Audits
- Bandit: A Python tool that scans for security issues in Python codebases.
- PyLint: A static code analysis tool that checks for potential security issues.
- OWASP ZAP: A security scanning tool that helps detect vulnerabilities in web applications.
9. Conclusion
Secure coding in Python is essential for developing robust applications that can withstand attacks and protect sensitive data. By following these best practices—such as input validation, secure authentication, and regular code reviews—you can reduce the risk of common vulnerabilities and build more resilient applications. Keep up-to-date with security trends and best practices, as the threat landscape continuously evolves.
Secure coding requires ongoing diligence and a proactive approach, so integrating these principles into your development process will pay off in the long term by minimizing potential vulnerabilities and ensuring your Python applications are as secure as possible.