Python continues to be one of the most popular programming languages, beloved for its simplicity, readability, and versatility. With each new release, Python introduces features and enhancements that make it even more powerful and efficient. The release of Python 3.12.3 is no exception. This comprehensive guide will explore the new features, improvements, and changes introduced in Python 3.12.3, providing you with a detailed understanding of how this update can benefit your development projects.
Overview of Python 3.12.3
Python 3.12.3 builds on the strengths of its predecessors, offering significant performance improvements, new language features, enhanced standard library modules, and important bug fixes. This release focuses on making Python faster, more secure, and more user-friendly.
Performance Improvements
1. Enhanced Interpreter Performance
Python 3.12.3 introduces several optimizations to the interpreter, resulting in improved performance for a wide range of applications. These enhancements include better handling of common operations and more efficient memory management.
Key Improvements:
- Bytecode Execution: Optimizations in the bytecode execution process lead to faster function calls and reduced overhead.
- Garbage Collection: Improvements in the garbage collector reduce pause times and enhance memory management efficiency.
2. Faster Built-in Functions
Several built-in functions have been optimized in Python 3.12.3, resulting in faster execution times. These optimizations are particularly noticeable in functions frequently used in data processing and numerical computations.
Examples:
- sum(): The
sum()
function has been optimized to handle large lists more efficiently. - min() and max(): Optimizations to
min()
andmax()
functions improve their performance when working with large datasets.
New Language Features
1. Match-Case Enhancements
Pattern matching, introduced in Python 3.10, has been further enhanced in Python 3.12.3. The match-case
syntax now supports more complex patterns, making it even more powerful and expressive.
Example:
1 2 3 4 5 6 7 8 9 10 11 | def process_data(data): match data: case {'type': 'text', 'content': str(content)}: print(f"Text content: {content}") case {'type': 'image', 'url': str(url)}: print(f"Image URL: {url}") case _: print("Unknown data type") data = {'type': 'text', 'content': 'Hello, world!'} process_data(data) |
These enhancements allow developers to write cleaner and more maintainable code when dealing with complex data structures.
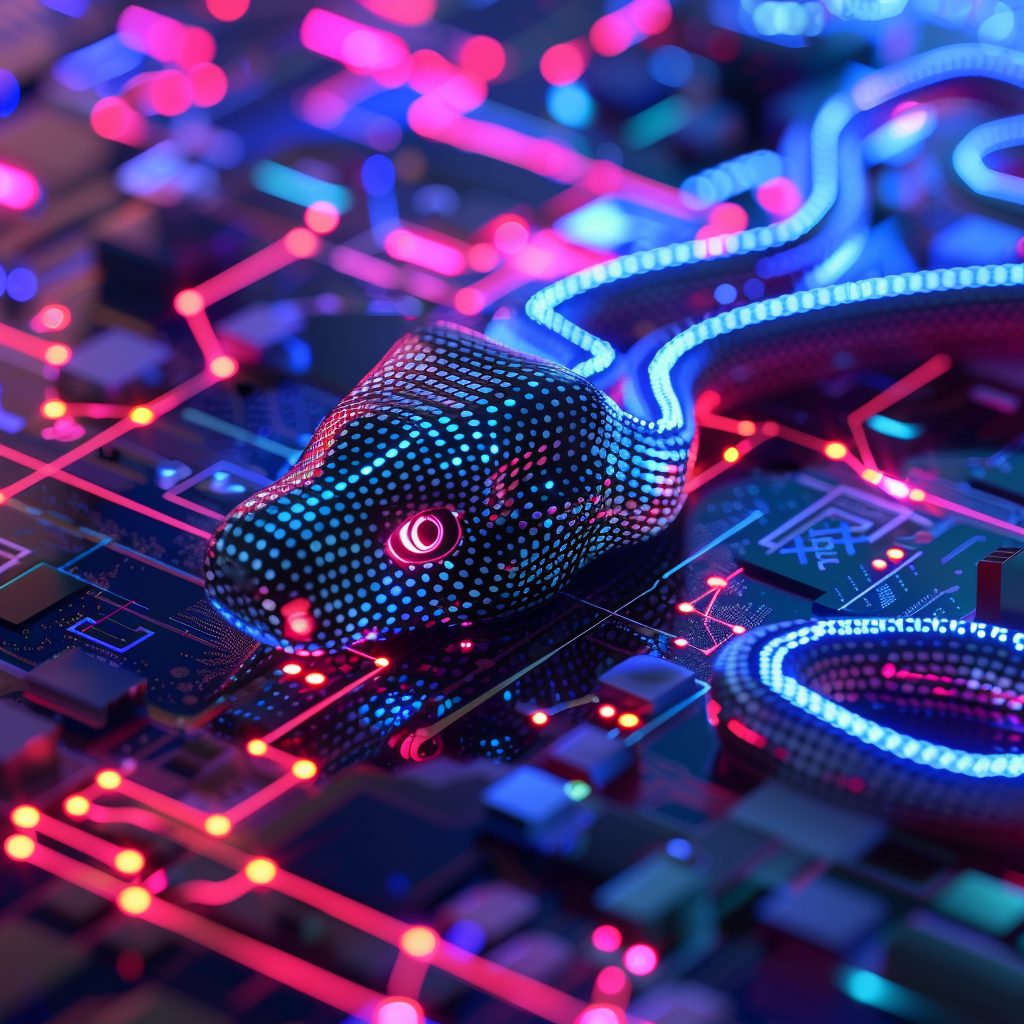
2. Improved Type Hints
Python 3.12.3 includes improvements to type hinting, making it easier to write and understand type annotations. These enhancements include better support for generic types and more precise type inference.
Example:
1 2 3 4 5 6 7 8 | from typing import List, Tuple def process_items(items: List[Tuple[str, int]]) -> None: for item in items: print(f"Item: {item[0]}, Quantity: {item[1]}") items = [('apple', 10), ('banana', 5)] process_items(items) |
These improvements help developers catch type-related errors earlier in the development process, leading to more robust code.
Enhanced Standard Library
1. New Modules and Functions
Python 3.12.3 introduces several new modules and functions in the standard library, expanding the language’s capabilities and making it easier to perform common tasks.
Notable Additions:
- statistics: The
statistics
module has been expanded with new functions for advanced statistical analysis. - dataclasses: Enhancements to the
dataclasses
module make it easier to define and work with data classes.
Example:
1 2 3 4 | from statistics import mean, median data = [10, 20, 30, 40, 50] print(f"Mean: {mean(data)}, Median: {median(data)}") |
2. Enhancements to Existing Modules
Several existing modules have received significant updates in Python 3.12.3, adding new features and improving performance.
Notable Enhancements:
- json: The
json
module has been optimized for faster serialization and deserialization of JSON data. - http.client: Improvements to the
http.client
module enhance the performance and security of HTTP connections.
Example:
1 2 3 4 5 | import json data = {'name': 'Alice', 'age': 30, 'city': 'New York'} json_data = json.dumps(data) print(json_data) |
Security Improvements
1. Enhanced Security Features
Python 3.12.3 includes several security enhancements to protect against common vulnerabilities and attacks. These improvements make Python a more secure choice for developing web applications and other software.
Key Enhancements:
- SSL/TLS: Improvements to the SSL/TLS stack enhance the security of encrypted communications.
- Hashing Algorithms: Updates to the
hashlib
module provide better support for modern hashing algorithms.
Example:
1 2 3 4 5 | import hashlib password = 'secure_password' hash_object = hashlib.sha256(password.encode()) print(hash_object.hexdigest()) |
2. Secure Default Settings
Python 3.12.3 introduces more secure default settings for various modules and functions. These changes help developers write secure code without needing to manually configure security settings.
Example:
1 2 3 4 | import ssl context = ssl.create_default_context() print(context.protocol) |
Developer Productivity
1. Enhanced Tooling
Python 3.12.3 comes with enhanced tooling support, making it easier for developers to write, debug, and maintain their code. Improvements to the Python Development Kit (PDK) include updates to popular tools such as the Python compiler (pyc), Python Virtual Machine (PVM), and debugging tools.
Key Improvements:
- pyc Enhancements: Better error messages and improved compilation performance.
- PVM Improvements: Enhanced performance monitoring and debugging capabilities.
- Integrated Development Environment (IDE) Support: Improved support in popular IDEs like PyCharm, VS Code, and Jupyter Notebook.
2. Enhanced APIs
Several new APIs and enhancements to existing ones have been introduced in Python 3.12.3 to make development more efficient and expressive.
Notable Enhancements:
- Asyncio: Improved support for asynchronous programming, including new utilities for managing asynchronous tasks.
- Pathlib: Enhancements to the
pathlib
module make it easier to work with file system paths.
Example:
1 2 3 4 5 6 7 8 | import asyncio async def fetch_data(): print("Fetching data...") await asyncio.sleep(2) print("Data fetched") asyncio.run(fetch_data()) |
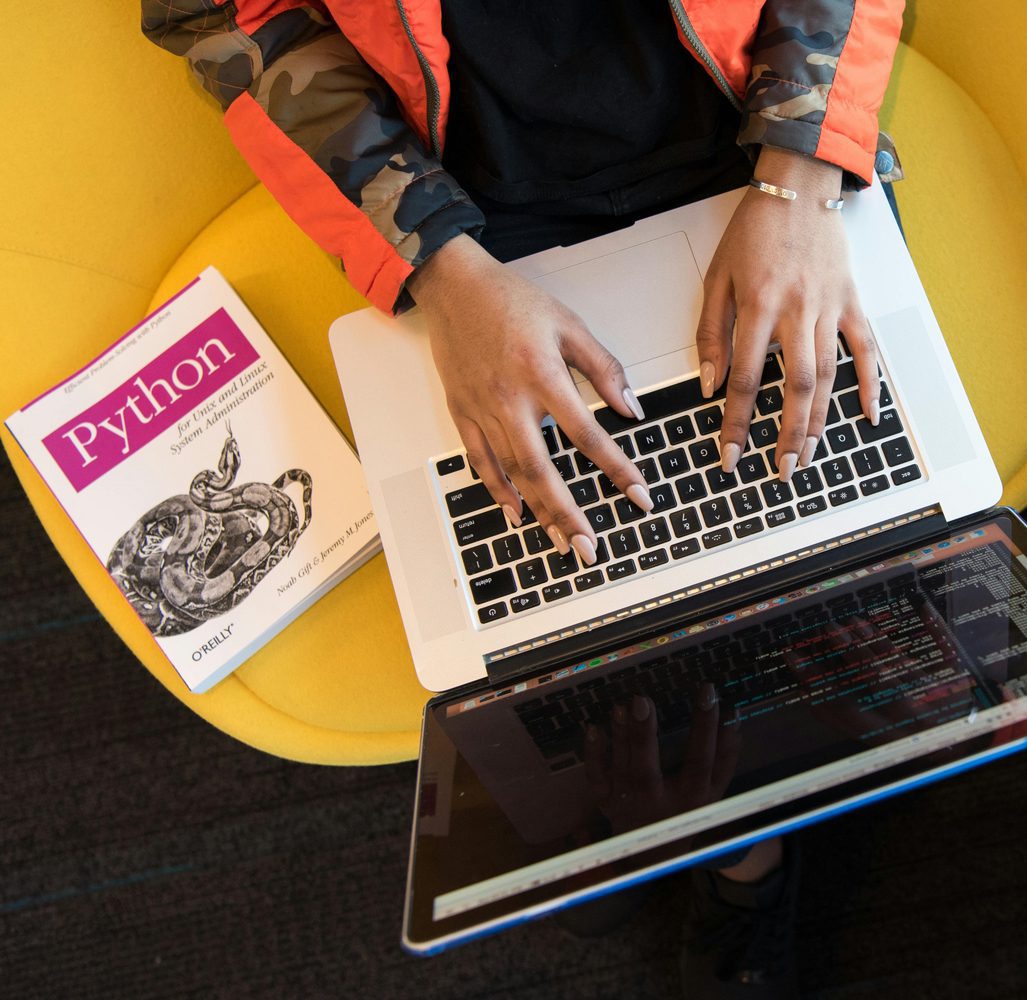
Community and Ecosystem
1. Open Source Contributions
Python 3.12.3 includes numerous contributions from the open-source community. These contributions come from a diverse set of individuals and organizations, highlighting the collaborative nature of Python’s development.
Notable Contributions:
- Performance Improvements: Contributions aimed at optimizing the performance of the Python interpreter and standard library.
- Bug Fixes: A large number of bug fixes and enhancements based on community feedback.
2. Ecosystem Growth
The Python ecosystem continues to grow, with a wide array of libraries, frameworks, and tools available to developers. Python 3.12.3 benefits from this rich ecosystem, offering better integration and support for modern development practices.
Popular Libraries and Frameworks:
- Django: Enhancements to support new Python 3.12.3 features.
- Flask: Improved integration with the latest Python features.
- NumPy/SciPy: Updated versions to leverage new performance improvements in Python 3.12.3.
Migration to Python 3.12.3
1. Preparing for Migration
Migrating to Python 3.12.3 involves several steps to ensure a smooth transition. Developers should start by reviewing the release notes and documentation to understand the changes and new features.
Migration Steps:
- Review Release Notes: Understand the new features and changes in Python 3.12.3.
- Update Dependencies: Ensure that all dependencies are compatible with Python 3.12.3.
- Run Tests: Thoroughly test applications to identify any issues or incompatibilities.
- Leverage New Features: Refactor code to take advantage of new features and improvements.
2. Compatibility Considerations
Python 3.12.3 maintains a high level of backward compatibility, but developers should be aware of any deprecated features and removed APIs. Reviewing the compatibility guide can help in identifying potential issues.
Key Considerations:
- Deprecated Features: Identify and replace any deprecated features.
- Removed APIs: Ensure that code does not rely on any removed APIs.
- Performance Testing: Conduct performance testing to ensure that the application benefits from the improvements in Python 3.12.3.
Conclusion
Python 3.12.3 represents a significant step forward in the evolution of the Python language. With its performance enhancements, new language features, improved security, and enhanced developer productivity, Python 3.12.3 is poised to empower developers to build more robust, efficient, and secure applications.
As you explore the new features and capabilities of Python 3.12.3, take advantage of the rich ecosystem of tools, libraries, and community resources available. Whether you are building web applications, data analysis tools, or scientific computing applications, Python 3.12.3 offers the features and performance you need to succeed.
Useful Links
By staying updated with the latest features and improvements in Python 3.12.3, you can ensure that your applications remain cutting-edge, efficient, and secure. Embrace the new capabilities of Python 3.12.3 and take your development projects to the next level. Stay tuned for more in-depth articles and tutorials on leveraging Python 3.12.3 for your development needs.