Writing clean code is an essential skill for any software developer. Clean code is not only easier to understand and maintain but also reduces the likelihood of bugs and improves overall software quality. In this comprehensive guide, we’ll explore best practices for writing clean code, focusing on principles that ensure maintainability, readability, and efficiency. By adhering to these practices, you can create software that stands the test of time, making life easier for both you and your team.
What is Clean Code?
Clean code refers to code that is easy to read, understand, and maintain. It follows established coding conventions, is well-documented, and minimizes complexity. Clean code makes it easier to identify and fix bugs, add new features, and understand the overall structure and flow of the program.
Key Characteristics of Clean Code:
- Readable: Code that can be easily understood by others.
- Maintainable: Code that is easy to modify and extend.
- Efficient: Code that performs well and uses resources wisely.
- Testable: Code that can be easily tested for correctness.
Principles of Clean Code
Meaningful Names
Choosing meaningful names for variables, functions, classes, and other entities is crucial for clean code. Good names convey intent and make the code self-explanatory.
Tips:
- Be Descriptive: Use names that describe the purpose and behavior of the entity.
- Use Pronounceable Names: Names should be easy to read and say out loud.
- Follow Naming Conventions: Adhere to established naming conventions for consistency.
Example: Instead of using variable names like x
or y
, use userName
or orderTotal
.
Functions Should Do One Thing
Functions should be designed to perform a single task or responsibility. This makes them easier to understand, test, and maintain.
Tips:
- Keep Functions Small: Aim for functions that are no longer than a few lines of code.
- Single Responsibility Principle: Each function should have only one reason to change.
- Use Descriptive Names: Function names should clearly indicate what they do.
Example: Instead of having a function that handles user login, loads user data, and updates the UI, break it into three separate functions.
Write Readable Code
Readable code is crucial for maintainability. Writing code that others can easily understand reduces the time needed for debugging and development.
Tips:
- Use Indentation and Spacing: Proper indentation and spacing make the code structure clear.
- Avoid Deep Nesting: Deeply nested code is harder to read and understand. Aim for a flat structure.
- Comment Wisely: Use comments to explain why something is done, not what is done. The code should be self-explanatory as much as possible.
Example: Use consistent indentation and add comments to explain complex logic.
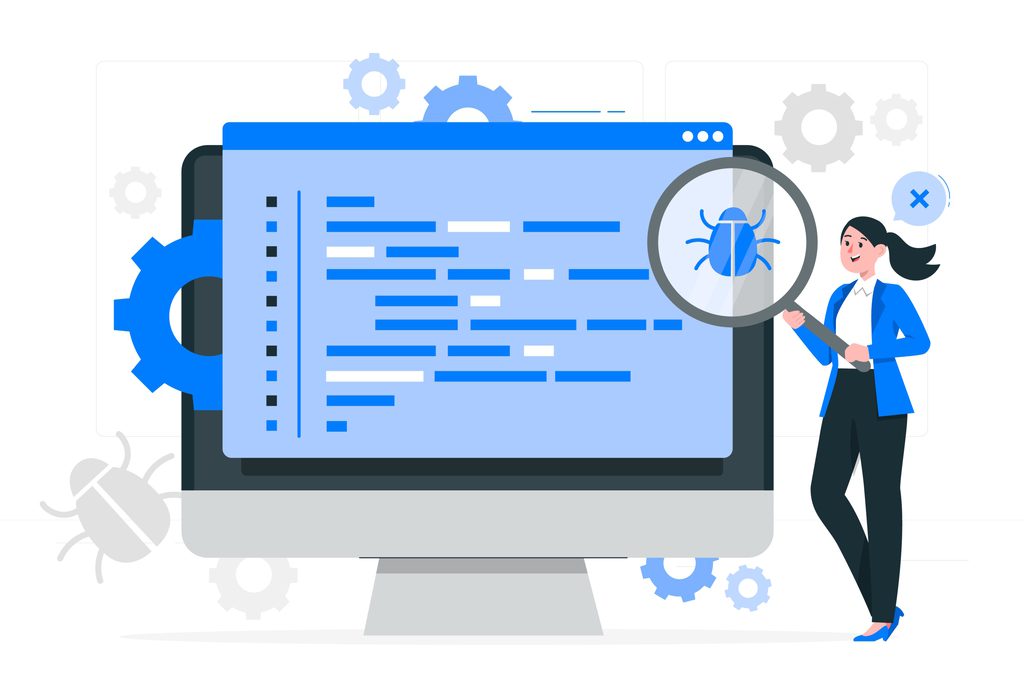
Best Practices for Writing Maintainable Software
Keep It Simple (KISS)
The KISS principle (Keep It Simple, Stupid) emphasizes simplicity in design and implementation. Simple code is easier to understand, test, and maintain.
Tips:
- Avoid Overengineering: Implement only what is necessary to meet the requirements.
- Use Simple Algorithms: Choose the simplest algorithm that solves the problem.
- Refactor Complex Code: Regularly refactor to simplify complex code.
DRY (Don’t Repeat Yourself)
The DRY principle advocates for reducing repetition in code. Repeated code can lead to inconsistencies and makes maintenance harder.
Tips:
- Use Functions and Classes: Encapsulate repeated logic in functions and classes.
- Refactor Common Patterns: Identify and refactor common patterns into reusable components.
- Avoid Magic Numbers: Replace repeated numbers or strings with named constants.
Write Tests
Writing tests ensures that your code works as expected and makes it easier to catch bugs early. It also facilitates refactoring and adding new features with confidence.
Types of Tests:
- Unit Tests: Test individual units of code (e.g., functions or classes) in isolation.
- Integration Tests: Test the interaction between different parts of the system.
- End-to-End Tests: Test the entire application workflow from start to finish.
Use Version Control
Version control systems (VCS) like Git help manage changes to code over time. They facilitate collaboration, track history, and enable safe experimentation.
Best Practices:
- Commit Frequently: Make small, incremental changes and commit them regularly.
- Write Descriptive Commit Messages: Clearly describe the changes made in each commit.
- Use Branching: Use branches to isolate development work, bug fixes, and features.
Code Review and Collaboration
Conduct Code Reviews
Code reviews involve examining code changes made by peers before merging them into the main codebase. They help identify potential issues and improve code quality.
Tips:
- Review for Logic and Style: Check both the logic and adherence to coding standards.
- Provide Constructive Feedback: Offer suggestions for improvement rather than just pointing out mistakes.
- Encourage Discussion: Use code reviews as an opportunity to discuss and learn from each other.
Use Collaborative Tools
Collaborative tools facilitate communication and coordination among team members, improving productivity and code quality.
Tools:
- Code Repositories: Platforms like GitHub and GitLab for hosting and managing code.
- Project Management: Tools like Jira and Trello for tracking tasks and progress.
- Communication: Tools like Slack and Microsoft Teams for real-time communication.
Documentation and Consistency
Document Your Code
Good documentation helps others understand your code and how to use it. It includes inline comments, README files, and API documentation.
Tips:
- Comment Purpose and Intent: Explain why the code exists and what it does.
- Maintain Up-to-Date Documentation: Ensure documentation reflects the current state of the code.
- Use Documentation Generators: Tools like Javadoc and Sphinx automate the creation of documentation.
Maintain Consistency
Consistency in coding style and practices reduces confusion and makes the codebase more cohesive and easier to manage.
Tips:
- Follow a Style Guide: Use established style guides for your programming language (e.g., PEP 8 for Python).
- Use Linters and Formatters: Tools like ESLint and Prettier enforce consistent style and formatting.
- Standardize Patterns and Practices: Agree on common patterns and practices for your team or project.
Advanced Techniques for Clean Code
Refactoring
Refactoring involves restructuring existing code without changing its external behavior. It improves code readability, maintainability, and performance.
Tips:
- Identify Code Smells: Look for signs of poor design, such as duplicated code and long methods.
- Refactor Regularly: Integrate refactoring into your development workflow.
- Use Automated Refactoring Tools: IDEs like IntelliJ IDEA and Eclipse provide tools for automated refactoring.
Design Patterns
Design patterns are proven solutions to common software design problems. They provide a shared vocabulary for developers and improve code reusability and flexibility.
Common Design Patterns:
- Creational Patterns: Deal with object creation mechanisms (e.g., Singleton, Factory).
- Structural Patterns: Deal with object composition (e.g., Adapter, Composite).
- Behavioral Patterns: Deal with object interaction and responsibility (e.g., Observer, Strategy).
Dependency Injection
Dependency Injection (DI) is a design pattern that helps achieve Inversion of Control (IoC) between classes and their dependencies. It improves code modularity and testability.
Benefits:
- Reduces Coupling: Classes are less dependent on specific implementations, making them easier to test and maintain.
- Improves Code Flexibility: Dependencies can be swapped out without changing the dependent class.
- Enhances Testability: Dependencies can be easily mocked or stubbed in tests.
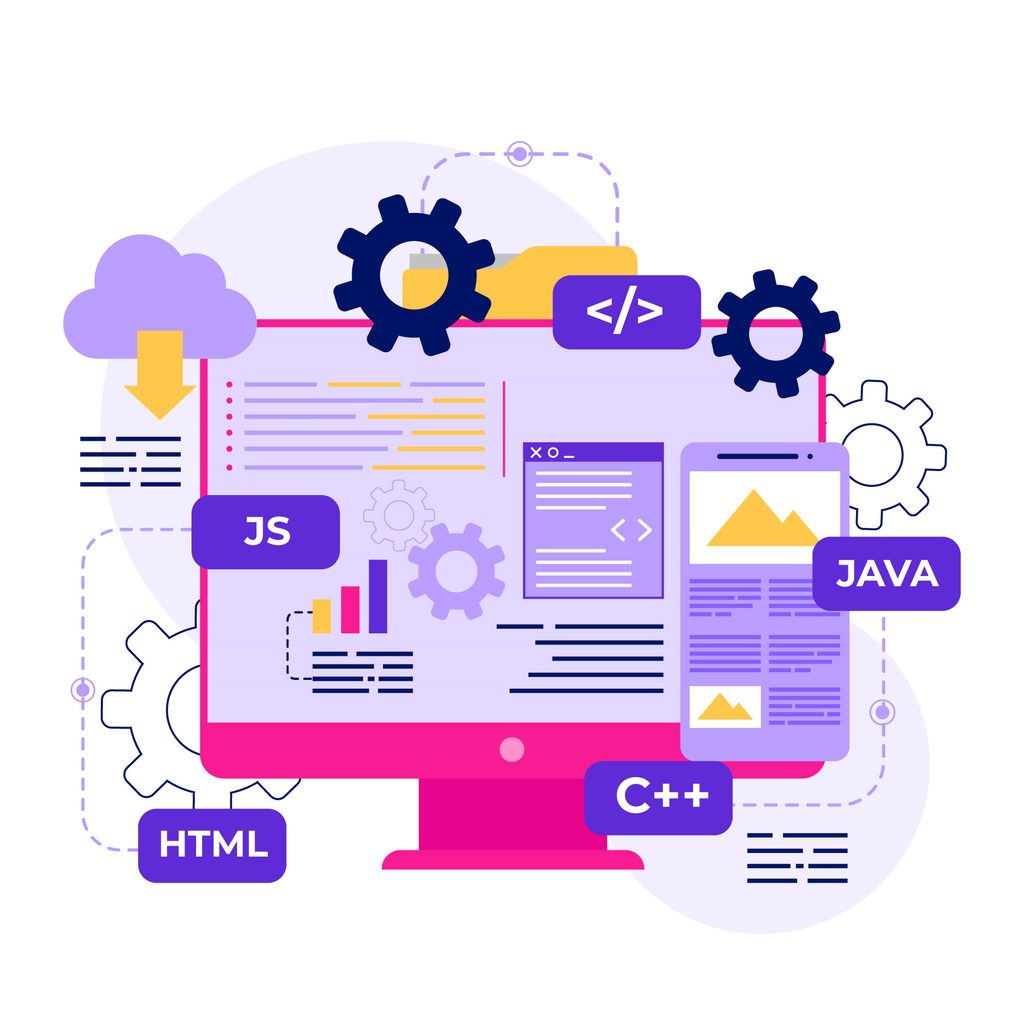
Practical Examples of Clean Code Practices
Example 1: Meaningful Names
Before:
1 2 3 4 | def func(a, b): return a + b x = func(5, 3) |
After:
1 2 3 4 | def add_numbers(number1, number2): return number1 + number2 sum_result = add_numbers(5, 3) |
Explanation: The function and variable names are more descriptive, making the code easier to understand.
Example 2: Single Responsibility Principle
Before:
1 2 3 4 5 6 7 8 9 10 11 12 13 | def process_order(order): validate_order(order) save_order(order) send_confirmation_email(order) def validate_order(order): # validation logic def save_order(order): # save logic def send_confirmation_email(order): # email logic |
After:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | class OrderProcessor: def __init__(self, order_validator, order_saver, email_sender): self.order_validator = order_validator self.order_saver = order_saver self.email_sender = email_sender def process_order(self, order): self.order_validator.validate(order) self.order_saver.save(order) self.email_sender.send(order) class OrderValidator: def validate(self, order): # validation logic class OrderSaver: def save(self, order): # save logic class EmailSender: def send(self, order): # email logic |
Explanation: The responsibilities are separated into different classes, making the code more modular and easier to maintain.
Example 3: Refactoring
Before:
1 2 3 4 5 6 7 | def calculate_discount(price, discount_type): if discount_type == 'student': return price * 0.9 elif discount_type == 'senior': return price * 0.8 else: return price |
After:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | class DiscountStrategy: def calculate(self, price): raise NotImplementedError() class StudentDiscount(DiscountStrategy): def calculate(self, price): return price * 0.9 class SeniorDiscount(DiscountStrategy): def calculate(self, price): return price * 0.8 class NoDiscount(DiscountStrategy): def calculate(self, price): return price def calculate_discount(price, discount_strategy): return discount_strategy.calculate(price) |
Explanation: The code is refactored using the Strategy Pattern, making it more flexible and easier to extend with new discount types.
Conclusion
Writing clean code is a fundamental skill for software developers. By following best practices such as using meaningful names, adhering to the single responsibility principle, keeping code simple, avoiding repetition, writing tests, and using version control, you can create maintainable and readable code. Additionally, conducting code reviews, documenting your code, maintaining consistency, and employing advanced techniques like refactoring, design patterns, and dependency injection can further enhance the quality of your software.
By prioritizing clean code, you not only improve your own productivity but also contribute to a more collaborative and efficient development environment. Embrace these best practices and make clean code an integral part of your coding philosophy.
Useful Links
- Clean Code Overview
- Naming Conventions in Code
- Single Responsibility Principle
- Writing Readable Code
- KISS Principle
- DRY Principle
- Writing Effective Unit Tests
- Git Best Practices
- Effective Code Review Practices
- Top Collaborative Tools for Developers
- Writing Good Documentation
- Importance of Consistency in Code
- Refactoring Techniques
- Introduction to Design Patterns
- Understanding Dependency Injection
By following this guide and exploring the resources provided, you can enhance your coding practices and create software that is clean, maintainable, and efficient. Happy coding!