In the world of software development, two of the most commonly used programming paradigms are Functional Programming (FP) and Object-Oriented Programming (OOP). Each approach offers a different way of thinking about code organization, problem-solving, and the flow of data within an application. While both paradigms are powerful and widely used, they are fundamentally different in how they approach structuring programs.
In this guide, we’ll break down the core concepts behind functional and object-oriented programming, compare their differences, and discuss their respective advantages and use cases. Whether you’re new to programming or trying to expand your skill set, understanding these two paradigms will help you choose the best approach for your projects.
Table of Contents
- What is Functional Programming?
- What is Object-Oriented Programming?
- Key Differences Between Functional and Object-Oriented Programming
- Core Principles of Functional Programming
- Core Principles of Object-Oriented Programming
- When to Use Functional Programming
- When to Use Object-Oriented Programming
- Real-World Examples and Use Cases
- Which Paradigm Should You Choose?
- Conclusion
1. What is Functional Programming?
Functional Programming (FP) is a programming paradigm that treats computation as the evaluation of mathematical functions. In FP, functions are the primary building blocks of a program, and they are treated as “first-class citizens.” This means that functions can be assigned to variables, passed as arguments to other functions, and returned from other functions.
Functional programming emphasizes immutability, pure functions, and higher-order functions. The goal of FP is to write programs that are declarative, where the code describes what should be done rather than how it should be done. This contrasts with imperative programming, where the focus is on explicitly defining the steps or procedures to achieve a task.
Key Characteristics of Functional Programming:
- Pure Functions: Functions that always produce the same output for the same input and have no side effects (i.e., they don’t alter global state or change variables outside their scope).
- Immutability: Data in FP is immutable, meaning once a value is assigned, it cannot be changed. This reduces unintended side effects and makes code easier to reason about.
- First-Class Functions: Functions can be assigned to variables, passed as arguments, and returned from other functions.
- Higher-Order Functions: Functions that can take other functions as arguments or return them as results.
- Declarative Programming: Focuses on describing the logic of the computation without specifying its control flow.
Languages that support functional programming include Haskell, Erlang, Elixir, and Lisp. Many modern languages, such as JavaScript, Python, and Java, also support functional programming features.
2. What is Object-Oriented Programming?
Object-Oriented Programming (OOP) is a programming paradigm that models software around objects. Objects are instances of classes, which define both the data (attributes) and the behaviors (methods) that operate on that data. OOP is designed to model real-world entities in a way that makes it easier to manage and maintain complex systems.
In OOP, the main goal is to encapsulate data and behavior within objects, providing a clear structure for large applications. OOP is based on four core principles: encapsulation, inheritance, polymorphism, and abstraction. These principles encourage reusability, modularity, and organization in software design.
Key Characteristics of Object-Oriented Programming:
- Classes and Objects: A class defines a blueprint for creating objects, which are instances that hold both state (data) and behavior (methods).
- Encapsulation: Bundling of data and the methods that operate on that data within objects, hiding the internal state from outside interference.
- Inheritance: The ability of a class to inherit properties and methods from another class, promoting code reuse and hierarchical organization.
- Polymorphism: The ability for different objects to be treated as instances of the same class through interfaces or base classes, often leading to more flexible code.
- Abstraction: Hiding the complex implementation details and exposing only the necessary parts of an object.
OOP is used in languages such as Java, C++, Python, Ruby, and C#. It is widely used in enterprise software, game development, and large systems requiring modularity and reuse.
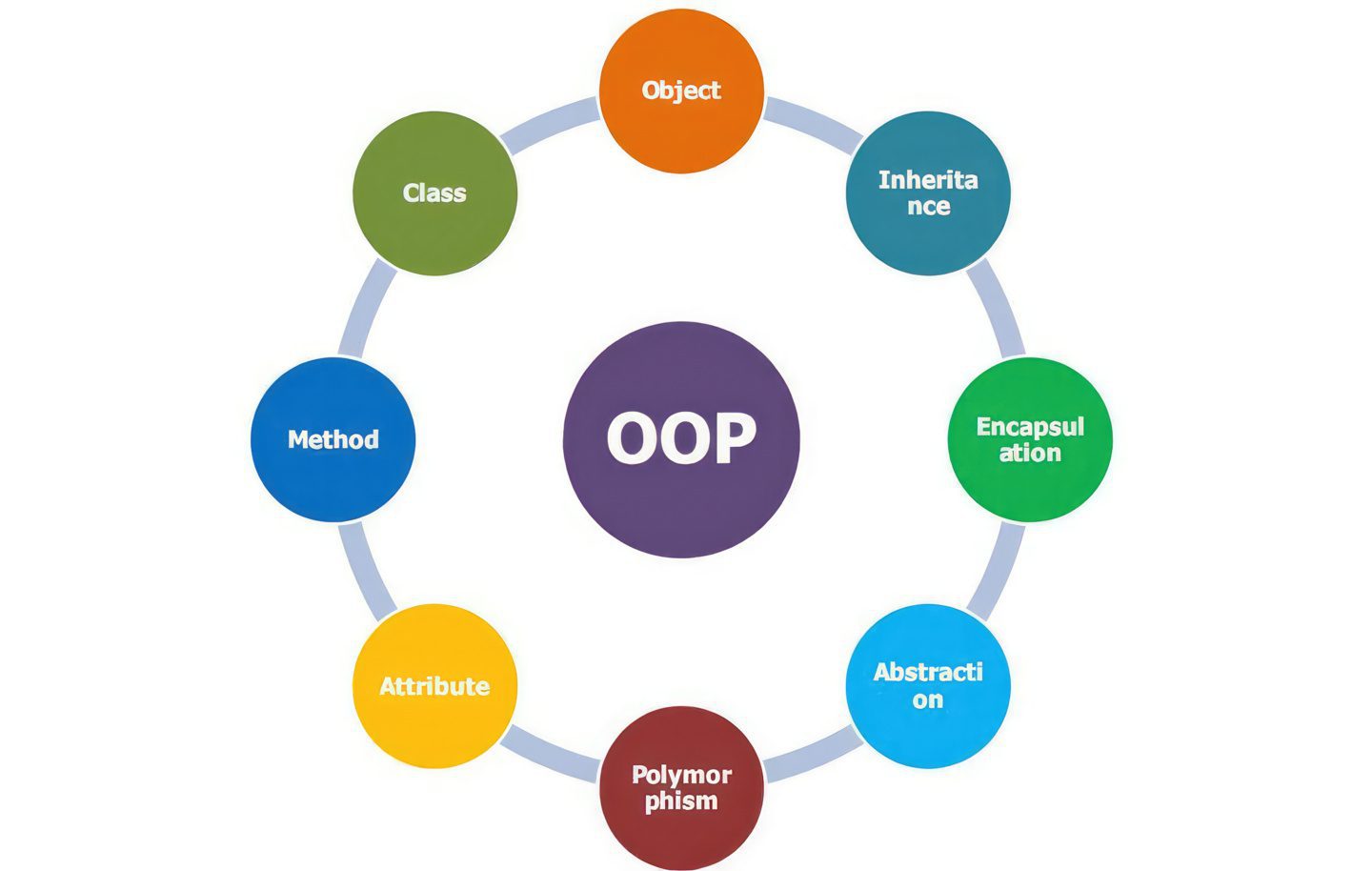
3. Key Differences Between Functional and Object-Oriented Programming
While both FP and OOP can be used to solve similar problems, they do so in fundamentally different ways. Here are the key differences between the two paradigms:
Feature | Functional Programming | Object-Oriented Programming |
---|---|---|
Core Concept | Functions and immutability | Objects and classes |
State Management | Stateless, relies on immutable data | Stateful, objects hold state and modify it over time |
Functions/Methods | First-class citizens, focus on pure functions | Methods belong to objects and operate on internal state |
Data Flow | Data flows through functions | Data is encapsulated within objects |
Reusability | Achieved through function composition and higher-order functions | Achieved through inheritance, polymorphism, and interfaces |
Mutability | Data is immutable | Data within objects can be mutable |
Side Effects | Avoids side effects, aims for pure functions | Methods can have side effects, including altering object state |
Type of Programming | Declarative | Imperative |
Concurrency Handling | Easier due to immutability and statelessness | Can be more complex due to shared mutable state |
4. Core Principles of Functional Programming
Functional programming is centered around several core principles that guide how programs are written and executed. Let’s take a closer look at these principles.
1. Pure Functions
Pure functions are the foundation of functional programming. A pure function is one that:
- Always produces the same output for the same input.
- Has no side effects (does not modify any external state or variables).
Pure functions are predictable, making them easier to test and debug. Since they don’t rely on external state, they can be reused without unintended consequences.
2. Immutability
In FP, once a value is assigned to a variable, it cannot be changed. This is known as immutability. Instead of modifying existing data, functions create and return new data structures. Immutability helps prevent bugs caused by unintended state changes and makes programs more predictable.
3. First-Class and Higher-Order Functions
In FP, functions are treated as first-class citizens, meaning they can be stored in variables, passed as arguments, and returned from other functions. Higher-order functions take other functions as arguments or return them as results. This leads to code that is more modular and reusable.
4. Function Composition
Function composition is a key concept in FP. It refers to the process of combining simple functions to build more complex functions. By composing functions together, you can create highly reusable and modular code. For example, you can chain multiple transformations on data by passing the result of one function to another.
5. Avoiding Side Effects
A side effect occurs when a function modifies some external state (e.g., updating a global variable or performing I/O operations). In FP, functions are designed to avoid side effects as much as possible. This leads to referential transparency, meaning that a function can be replaced with its output without changing the program’s behavior.
5. Core Principles of Object-Oriented Programming
Object-Oriented Programming follows a different set of core principles that are designed to promote modularity, code reuse, and organization.
1. Encapsulation
Encapsulation is the bundling of data (attributes) and the methods that operate on that data within an object. This hides the internal state of the object and only exposes a limited interface to interact with it. Encapsulation prevents external code from directly accessing and modifying the object’s state, reducing the likelihood of bugs.
2. Inheritance
Inheritance allows a class (subclass) to inherit properties and methods from another class (superclass). This promotes code reuse, as common functionality can be defined in a parent class and shared across multiple child classes. It also helps model real-world relationships, like a Car
class inheriting from a Vehicle
class.
3. Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables flexibility in code design, as you can write code that works with objects of different types without knowing their specific class. For example, a draw()
method can be called on different shapes (circle, square), and each shape can have its own implementation.
4. Abstraction
Abstraction is the process of simplifying complex systems by hiding the implementation details and exposing only the necessary parts. In OOP, this is achieved by defining abstract classes or interfaces that provide a high-level view of what an object can do, while hiding the specifics of how it does it. This allows developers to focus on “what” an object does rather than “how” it works.
5. Message Passing
In OOP, objects communicate with each other by sending and receiving messages (method calls). This interaction is central to the OOP model, where objects interact and collaborate to perform tasks.
Message passing allows objects to work together while maintaining their own state and behavior.
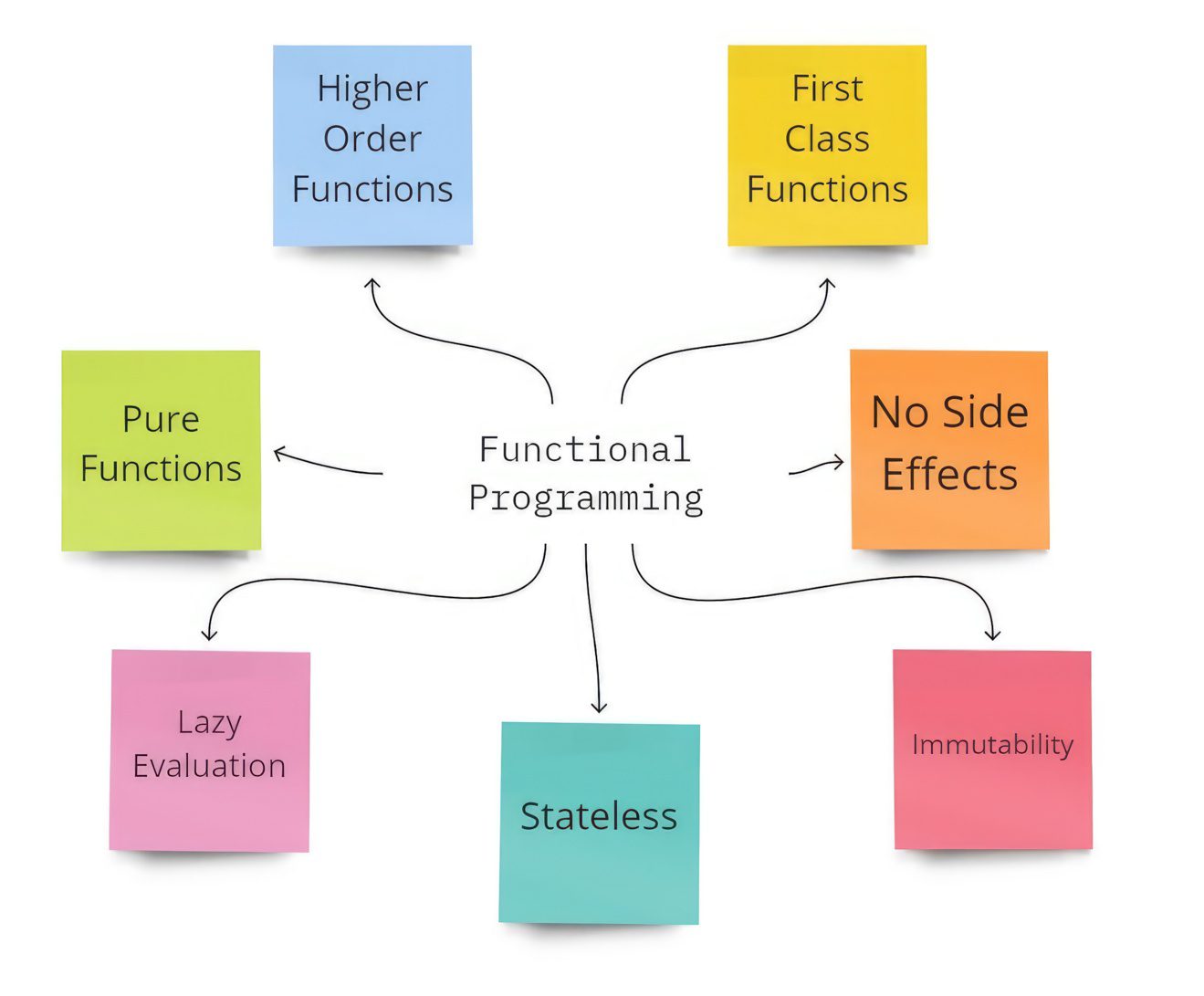
6. When to Use Functional Programming
Functional programming is best suited for situations where immutability, predictability, and modularity are important. Here are some cases where FP shines:
- Mathematical computations: FP’s emphasis on pure functions and immutability makes it a natural fit for tasks that involve mathematical operations and transformations.
- Data transformation pipelines: FP’s function composition and higher-order functions make it easy to create pipelines that process data in stages, transforming it step by step.
- Concurrency and parallelism: Immutability makes it easier to handle concurrency, as there is no need to worry about shared mutable state. This is particularly useful in multi-threaded or distributed systems.
- Declarative programming: If you want to write code that focuses on what the program should do, rather than how to do it, FP’s declarative nature is a good fit.
- Reactive programming: FP is often used in reactive programming, where applications respond to events and data streams (e.g., reactive JavaScript libraries like RxJS).
7. When to Use Object-Oriented Programming
Object-Oriented Programming is a great choice when you need to model real-world entities and maintain state across an application. Some use cases where OOP is particularly effective include:
- Large, complex systems: OOP is ideal for organizing and maintaining large codebases, where encapsulation, inheritance, and polymorphism provide structure and reusability.
- GUI applications: Many graphical user interfaces (GUIs) are built using OOP principles, where different objects (e.g., windows, buttons) have their own state and behavior.
- Game development: OOP is well-suited for modeling game objects, where entities like characters, weapons, and environments can be represented as objects with unique properties and behaviors.
- Enterprise applications: Large enterprise systems often involve complex workflows and data models, which can be effectively managed using OOP’s encapsulation and inheritance features.
- Software that mimics real-world entities: If you are building software that represents real-world objects (e.g., vehicles, employees, bank accounts), OOP’s class and object model maps well to these scenarios.
8. Real-World Examples and Use Cases
To better understand how FP and OOP can be applied, let’s look at some real-world examples and industries that make heavy use of each paradigm.
Functional Programming in Action:
- Data Processing: Languages like Scala and Haskell are often used in data processing pipelines where large datasets need to be transformed through various stages. The emphasis on immutability ensures that the data remains consistent and free from side effects.
- Finance: Functional programming is popular in financial industries, where calculations and algorithms need to be precise, predictable, and free from unintended state changes. Banks often use FP to handle transactions and pricing models.
- Machine Learning: FP principles are well-suited for the mathematical nature of machine learning. Libraries like TensorFlow (which supports Python’s functional features) help process data in a functional way.
Object-Oriented Programming in Action:
- Web Development: In web frameworks like Django (Python) and Ruby on Rails, OOP is used to model entities like users, posts, or products in e-commerce platforms. Each entity has its own class, properties, and methods.
- Mobile App Development: Mobile apps written in Swift (iOS) or Java/Kotlin (Android) often use OOP to model the different screens, buttons, and interactions that the app contains.
- Simulation Software: Simulations (such as those used in aerospace or engineering) often use OOP to model different components or environments. For example, a flight simulator might model various airplanes, weather conditions, and sensors as objects.
9. Which Paradigm Should You Choose?
There’s no one-size-fits-all answer when it comes to choosing between FP and OOP. Both paradigms have their advantages, and the best choice depends on your project’s requirements, team expertise, and the type of application you’re building.
- Choose Functional Programming if:
- You need to handle large datasets or perform complex data transformations.
- Predictability and immutability are crucial for your application (e.g., financial systems, scientific computing).
- You are building a concurrent or parallel system where shared mutable state would be problematic.
- Choose Object-Oriented Programming if:
- You are building a large, stateful application that will evolve over time.
- Your application mimics real-world entities (e.g., a product catalog, banking system, or employee management).
- You want to structure your code in a way that promotes code reuse through inheritance and polymorphism.
In practice, many modern programming languages support both paradigms to some extent. For example, JavaScript, Python, and C++ allow developers to mix functional and object-oriented styles. This means you don’t always have to choose one paradigm exclusively—often, a hybrid approach can give you the best of both worlds.
10. Conclusion
Functional programming and object-oriented programming are two distinct paradigms that offer different approaches to software development. While FP focuses on pure functions, immutability, and statelessness, OOP revolves around objects, encapsulation, and managing state. Each paradigm has its strengths and weaknesses, and choosing between them depends on the specific needs of your project.
Functional programming is ideal for tasks that require immutability, predictable results, and stateless computations. On the other hand, object-oriented programming is perfect for modeling real-world entities, managing complex state, and building large-scale applications. By understanding the core concepts of each paradigm, you can make better decisions about how to structure your code and choose the best approach for your project.
Ultimately, both FP and OOP are powerful tools, and the best programmers are those who can apply the right paradigm at the right time.
Join the Cosmic Meta Community!
Stay ahead of the tech curve with expert insights, cutting-edge trends, and practical tips delivered straight to your inbox. Subscribe now and unlock the power of the digital age!