Object-Oriented Programming (OOP) has become a cornerstone of modern software development, offering a powerful paradigm for organizing and structuring code. By encapsulating data and behavior into objects, OOP facilitates code reuse, scalability, and maintainability. This comprehensive guide will delve into the fundamentals of OOP, its key principles, advantages, and practical applications. Whether you’re a seasoned developer or just starting out, this post aims to provide a deep understanding of OOP and its significance in the programming world.
What is Object-Oriented Programming?
Definition of Object-Oriented Programming
Object-Oriented Programming (OOP) is a programming paradigm that uses objects to represent data and methods to manipulate that data. An object is an instance of a class, which can be thought of as a blueprint for creating objects. OOP focuses on defining the data structures (objects) and the operations (methods) that can be performed on them.
Key Principles of Object-Oriented Programming
OOP is based on four main principles:
- Encapsulation: Encapsulation involves bundling the data (attributes) and the methods (functions) that operate on the data into a single unit, or class. This principle restricts direct access to some of the object’s components, which can help prevent unintended interference and misuse.
- Abstraction: Abstraction means hiding the complex implementation details and showing only the essential features of the object. This simplifies the interaction with objects by exposing only the necessary functionalities.
- Inheritance: Inheritance is a mechanism by which one class (child or subclass) inherits the attributes and methods of another class (parent or superclass). This promotes code reuse and the creation of hierarchical relationships between classes.
- Polymorphism: Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables a single interface to represent different underlying forms (data types).
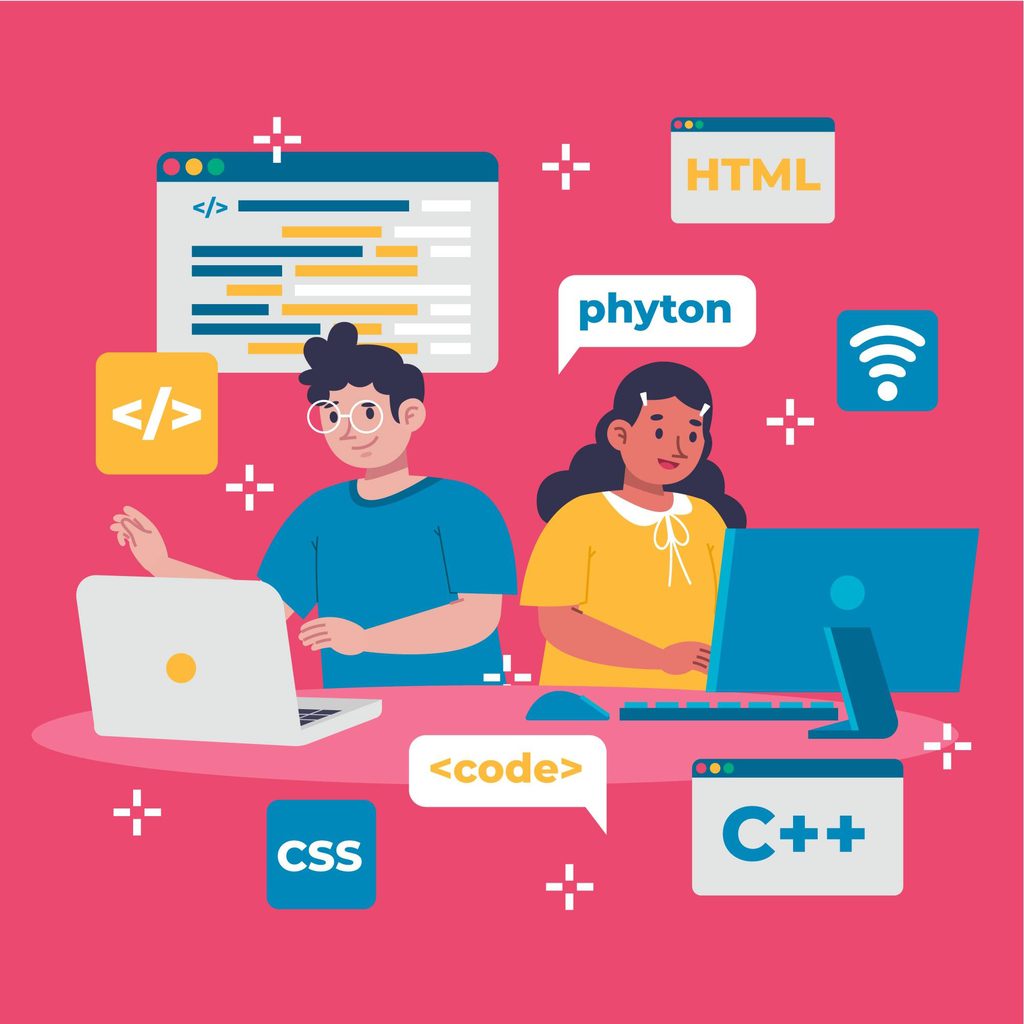
The Evolution of Object-Oriented Programming
Historical Background
OOP has its roots in the 1960s, with the development of the Simula language by Ole-Johan Dahl and Kristen Nygaard. Simula was designed for simulations and introduced the concept of classes and objects. The principles of OOP were further refined and popularized by languages such as Smalltalk, developed in the 1970s by Alan Kay and his team at Xerox PARC.
The Rise of OOP Languages
The 1980s and 1990s saw the emergence of several OOP languages that have become mainstays in the programming world:
- C++: An extension of the C language that introduced classes and objects, making it one of the first widely adopted OOP languages.
- Java: Developed by Sun Microsystems, Java emphasized portability and ease of use, quickly becoming popular for enterprise applications.
- Python: Known for its simplicity and readability, Python supports multiple programming paradigms, including OOP.
- Ruby: Designed for productivity and fun, Ruby is an OOP language that powers the Ruby on Rails web framework.
Key Concepts in Object-Oriented Programming
To fully understand OOP, it’s essential to grasp the key concepts that underpin this programming paradigm.
Classes and Objects
Classes: A class is a blueprint for creating objects. It defines the attributes (data) and methods (functions) that the objects created from the class will have.
Objects: An object is an instance of a class. It represents a specific entity with attributes and methods defined by its class.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | class Car: def __init__(self, make, model, year): self.make = make self.model = model self.year = year def display_info(self): print(f"{self.year} {self.make} {self.model}") # Creating objects # Accessing object methods car1.display_info() # Output: 2020 Toyota Camry car2.display_info() # Output: 2019 Honda Accord |
Encapsulation
Encapsulation is the practice of keeping the data (attributes) and the code (methods) that manipulates the data together in one unit (class). It restricts direct access to some of an object’s components, which is a means of preventing unintended interference and misuse.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | class Account: def __init__(self, owner, balance=0): self.owner = owner self.__balance = balance def deposit(self, amount): self.__balance += amount print(f"{amount} deposited. New balance: {self.__balance}") def withdraw(self, amount): if amount > self.__balance: print("Insufficient funds") else: self.__balance -= amount print(f"{amount} withdrawn. New balance: {self.__balance}") # Creating an account object acc = Account("John Doe", 100) # Accessing methods acc.deposit(50) # Output: 50 deposited. New balance: 150 acc.withdraw(75) # Output: 75 withdrawn. New balance: 75 |
Abstraction
Abstraction is the concept of hiding the complex implementation details and showing only the essential features of the object. It simplifies the interaction with objects by exposing only the necessary functionalities.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | from abc import ABC, abstractmethod class Animal(ABC): @abstractmethod def sound(self): pass class Dog(Animal): def sound(self): return "Bark" class Cat(Animal): def sound(self): return "Meow" # Creating objects dog = Dog() cat = Cat() # Accessing methods print(dog.sound()) # Output: Bark print(cat.sound()) # Output: Meow |
Inheritance
Inheritance allows a class (child class) to inherit attributes and methods from another class (parent class). This promotes code reuse and establishes a hierarchical relationship between classes.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | class Vehicle: def __init__(self, brand, model): self.brand = brand self.model = model def display_info(self): print(f"{self.brand} {self.model}") class Car(Vehicle): def __init__(self, brand, model, doors): super().__init__(brand, model) self.doors = doors def display_info(self): super().display_info() print(f"Doors: {self.doors}") # Creating an object # Accessing methods car.display_info() # Output: # Tesla Model S # Doors: 4 |
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables a single interface to represent different underlying forms (data types).
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | class Bird: def fly(self): print("Flying...") class Sparrow(Bird): def fly(self): print("Sparrow flying...") class Eagle(Bird): def fly(self): print("Eagle flying...") # Function that takes a Bird object def make_bird_fly(bird): bird.fly() # Creating objects sparrow = Sparrow() eagle = Eagle() # Accessing methods make_bird_fly(sparrow) # Output: Sparrow flying... make_bird_fly(eagle) # Output: Eagle flying... |
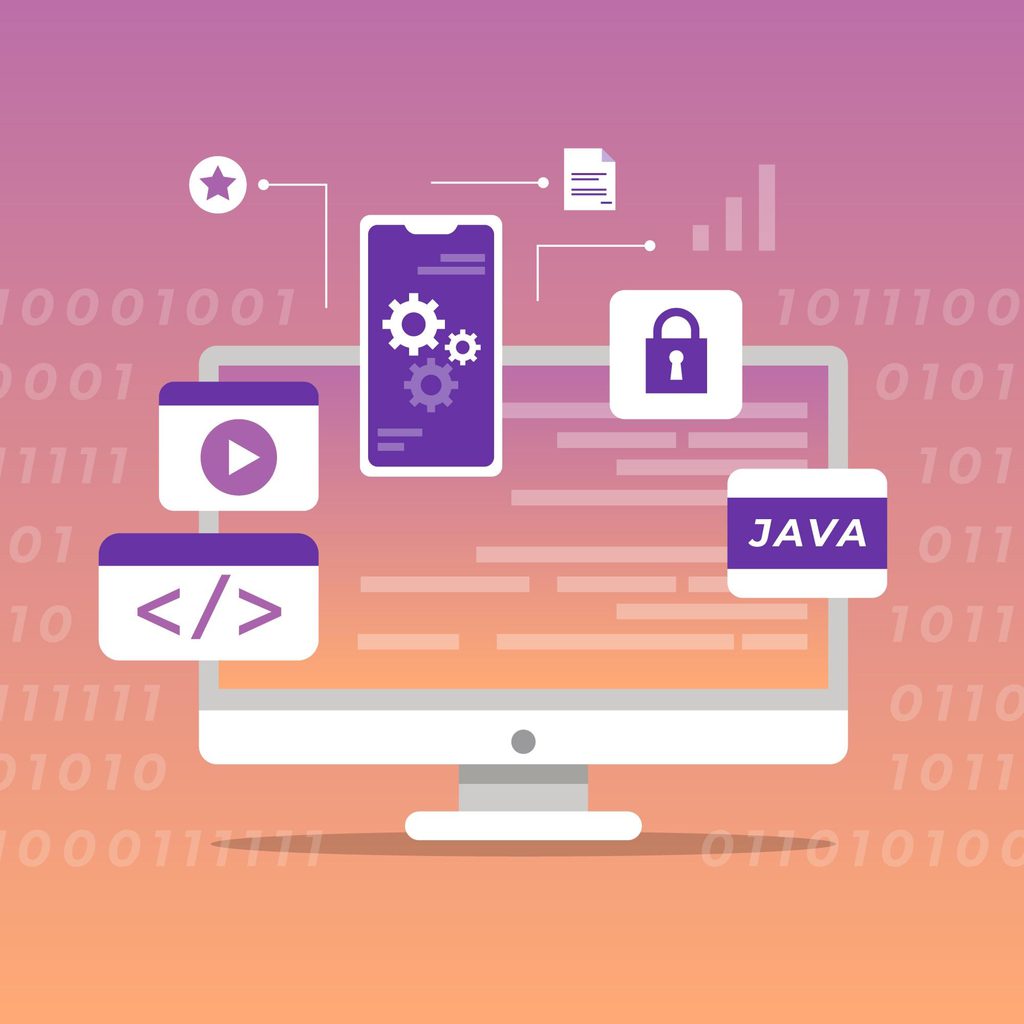
Advantages of Object-Oriented Programming
Object-Oriented Programming offers several advantages that make it a popular choice for software development.
1. Code Reusability
OOP promotes code reusability through inheritance and polymorphism. By defining common attributes and methods in a base class, developers can create new classes that inherit and extend these features. This reduces code duplication and promotes the use of tested and reliable code components.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | class Animal: def __init__(self, name): self.name = name def speak(self): pass class Dog(Animal): def speak(self): return "Bark" class Cat(Animal): def speak(self): return "Meow" # Creating objects dog = Dog("Buddy") cat = Cat("Whiskers") # Accessing methods print(dog.speak()) # Output: Bark print(cat.speak()) # Output: Meow |
2. Scalability and Maintainability
OOP makes it easier to scale and maintain code by organizing it into modular, self-contained units (classes). Each class can be developed, tested, and debugged independently, making the codebase more manageable.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | class Library: def __init__(self): self.books = [] def add_book(self, book): self.books.append(book) def remove_book(self, book): self.books.remove(book) def list_books(self): for book in self.books: print(book) class Book: def __init__(self, title, author): self.title = title self.author = author def __str__(self): return f"{self.title} by {self.author}" # Creating objects library = Library() book1 = Book("1984", "George Orwell") book2 = Book("To Kill a Mockingbird", "Harper Lee") # Using methods library.add_book(book1) library.add_book(book2) library.list_books() # Output: # 1984 by George Orwell # To Kill a Mockingbird by Harper Lee |
3. Improved Productivity
OOP enhances productivity by providing a clear structure for code, enabling developers to work more efficiently. The use of classes and objects allows for easier management of large codebases and simplifies debugging and testing processes.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | class Calculator: return x + y def subtract(self, x, y): return x - y def multiply(self, x, y): return x * y def divide(self, x, y): if y != 0: return x / y else: return "Error: Division by zero" # Creating an object calc = Calculator() # Using methods print(calc.add(5, 3)) # Output: 8 print(calc.subtract(5, 3)) # Output: 2 print(calc.multiply(5, 3)) # Output: 15 print(calc.divide(5, 3)) # Output: 1.6666666666666667 print(calc.divide(5, 0)) # Output: Error: Division by zero |
4. Enhanced Collaboration
OOP facilitates collaboration among developers by providing a clear framework for dividing work. Different developers can work on different classes or components simultaneously, ensuring that the overall development process is more efficient and organized.
Example:
In a team project, one developer might focus on creating a class for handling user authentication, while another works on a class for managing database interactions. By clearly defining the roles and responsibilities of each class, the team can work more cohesively.
Real-World Applications of Object-Oriented Programming
OOP is widely used in various industries and applications, demonstrating its versatility and effectiveness.
1. Web Development
OOP is commonly used in web development frameworks to build robust and scalable web applications. For example, frameworks like Django (Python), Ruby on Rails (Ruby), and Spring (Java) leverage OOP principles to provide reusable components and maintainable codebases.
Example:
In Django, models, views, and templates are organized as classes, making it easier to manage and extend web applications.
2. Game Development
Game development often involves complex interactions and behaviors, making OOP an ideal paradigm. Game objects, such as characters, enemies, and items, are represented as classes with attributes and methods defining their behaviors.
Example:
Unity, a popular game development engine, uses C# and OOP principles to create interactive and immersive game experiences.
3. Software Engineering
OOP is a fundamental paradigm in software engineering, used to develop a wide range of software products, from operating systems to enterprise applications. The modular nature of OOP makes it easier to manage large codebases and implement changes over time.
Example:
Operating systems like Windows and macOS are developed using OOP languages like C++ and Objective-C, ensuring a maintainable and scalable codebase.
Challenges and Criticisms of Object-Oriented Programming
While OOP offers numerous benefits, it also faces certain challenges and criticisms.
1. Complexity
OOP can introduce additional complexity, especially for beginners. Understanding concepts like inheritance, polymorphism, and encapsulation can be challenging and may lead to over-engineering if not used appropriately.
2. Performance Overhead
OOP languages often introduce performance overhead due to features like dynamic dispatch, object creation, and garbage collection. In performance-critical applications, this overhead can be a concern.
Example:
In high-frequency trading systems or real-time applications, developers might prefer procedural or functional programming languages to minimize performance overhead.
3. Misuse of Inheritance
Improper use of inheritance can lead to tightly coupled code and maintenance challenges. Overusing inheritance can create complex hierarchies that are difficult to understand and modify.
Solution:
Favor composition over inheritance by building classes from components rather than extending them. This approach promotes flexibility and code reuse without the pitfalls of deep inheritance hierarchies.
Best Practices for Object-Oriented Programming
To effectively use OOP, it is essential to follow best practices that promote clean, maintainable, and efficient code.
1. Follow SOLID Principles
The SOLID principles are a set of design principles that help developers create flexible and maintainable software.
- Single Responsibility Principle: A class should have only one reason to change, meaning it should have only one job.
- Open/Closed Principle: Classes should be open for extension but closed for modification.
- Liskov Substitution Principle: Subtypes should be substitutable for their base types without altering the correctness of the program.
- Interface Segregation Principle: Clients should not be forced to depend on interfaces they do not use.
- Dependency Inversion Principle: High-level modules should not depend on low-level modules; both should depend on abstractions.
Example:
Applying SOLID principles helps create a more modular and testable codebase, making it easier to manage and extend.
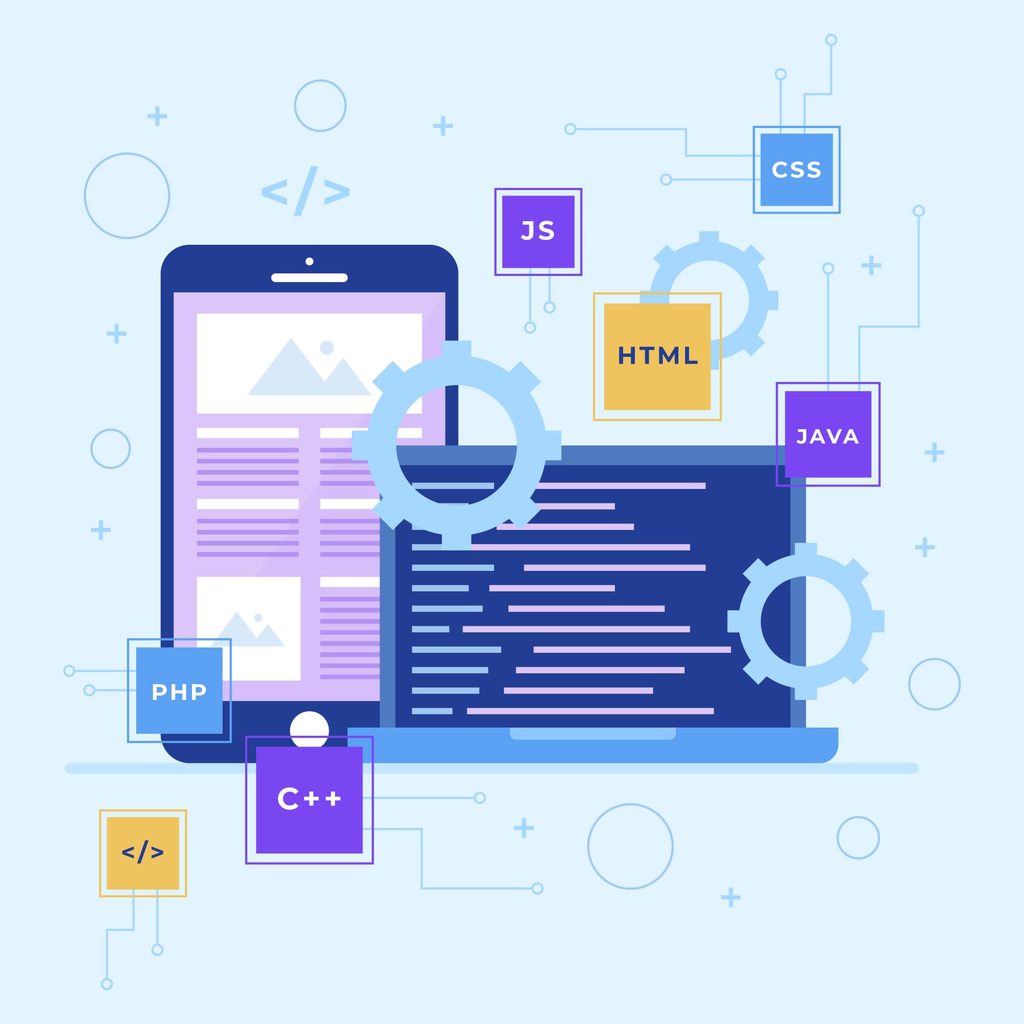
2. Use Design Patterns
Design patterns are proven solutions to common design problems. Familiarity with design patterns can help developers address recurring issues and improve code quality.
Examples of Design Patterns:
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
- Factory Method: Defines an interface for creating objects but lets subclasses alter the type of objects that will be created.
- Observer: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
Example:
Using the Singleton pattern to manage a database connection in an application ensures that only one instance of the connection is created, reducing resource usage and potential conflicts.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | class DatabaseConnection: _instance = None def __new__(cls): if cls._instance is None: cls._instance = super(DatabaseConnection, cls).__new__(cls) return cls._instance def connect(self): print("Connecting to the database...") # Using the singleton db1 = DatabaseConnection() db2 = DatabaseConnection() print(db1 == db2) # Output: True |
3. Write Clean and Readable Code
Maintaining clean and readable code is essential for collaboration and long-term maintenance. Use meaningful variable and method names, adhere to a consistent coding style, and document your code effectively.
Conclusion
Object-Oriented Programming (OOP) has revolutionized the way we develop software, offering a powerful and flexible paradigm for structuring code. By encapsulating data and behavior into objects, OOP promotes code reuse, scalability, and maintainability. Understanding the key principles of OOP—encapsulation, abstraction, inheritance, and polymorphism—along with best practices, can help developers create robust and efficient software solutions.
As OOP continues to evolve and adapt to new challenges and technologies, it remains a fundamental approach in the world of software development. By mastering OOP, developers can build high-quality software that meets the needs of users and businesses alike.
Useful Links
- Python Object-Oriented Programming
- Java Object-Oriented Programming Concepts
- C++ Object-Oriented Programming
- Design Patterns in Object-Oriented Programming
- SOLID Principles
By diving deep into the concepts, advantages, and real-world applications of Object-Oriented Programming, this guide aims to provide a thorough understanding of OOP and its significance in modern software development. Stay tuned for more in-depth articles and updates on programming paradigms and best practices.