In the ever-evolving world of software development, writing clean, maintainable, and scalable code is not just a best practice—it’s a fundamental necessity. As projects grow in size and complexity, and as development teams scale across organizations, following proven design patterns helps ensure consistency, clarity, and quality.
However, lurking alongside these best practices are their notorious counterparts: anti-patterns. These are common programming habits that may seem convenient or harmless in the short term but ultimately create confusion, inefficiency, and instability within codebases. Often the result of time pressure, inexperience, or technical debt, anti-patterns are deceptive—they give the illusion of progress while quietly undermining long-term maintainability.
The good news? Anti-patterns can be identified, refactored, and avoided. Whether you’re a new developer learning foundational skills or a seasoned engineer leading architecture discussions, understanding these pitfalls will help you write better, cleaner code.
Below, we explore ten of the most problematic programming anti-patterns, why they matter, and how to fix them.
1. God Object
A God Object is a class that tries to do everything. It often contains excessive responsibilities, making it the central hub of your code—but in all the wrong ways.
Why it’s bad: It violates the Single Responsibility Principle, making the code difficult to test, extend, or maintain. Any change becomes risky and error-prone.
How to fix it: Refactor the God Object into multiple, smaller components or services. Assign a single responsibility to each class and use well-defined interfaces to decouple logic.
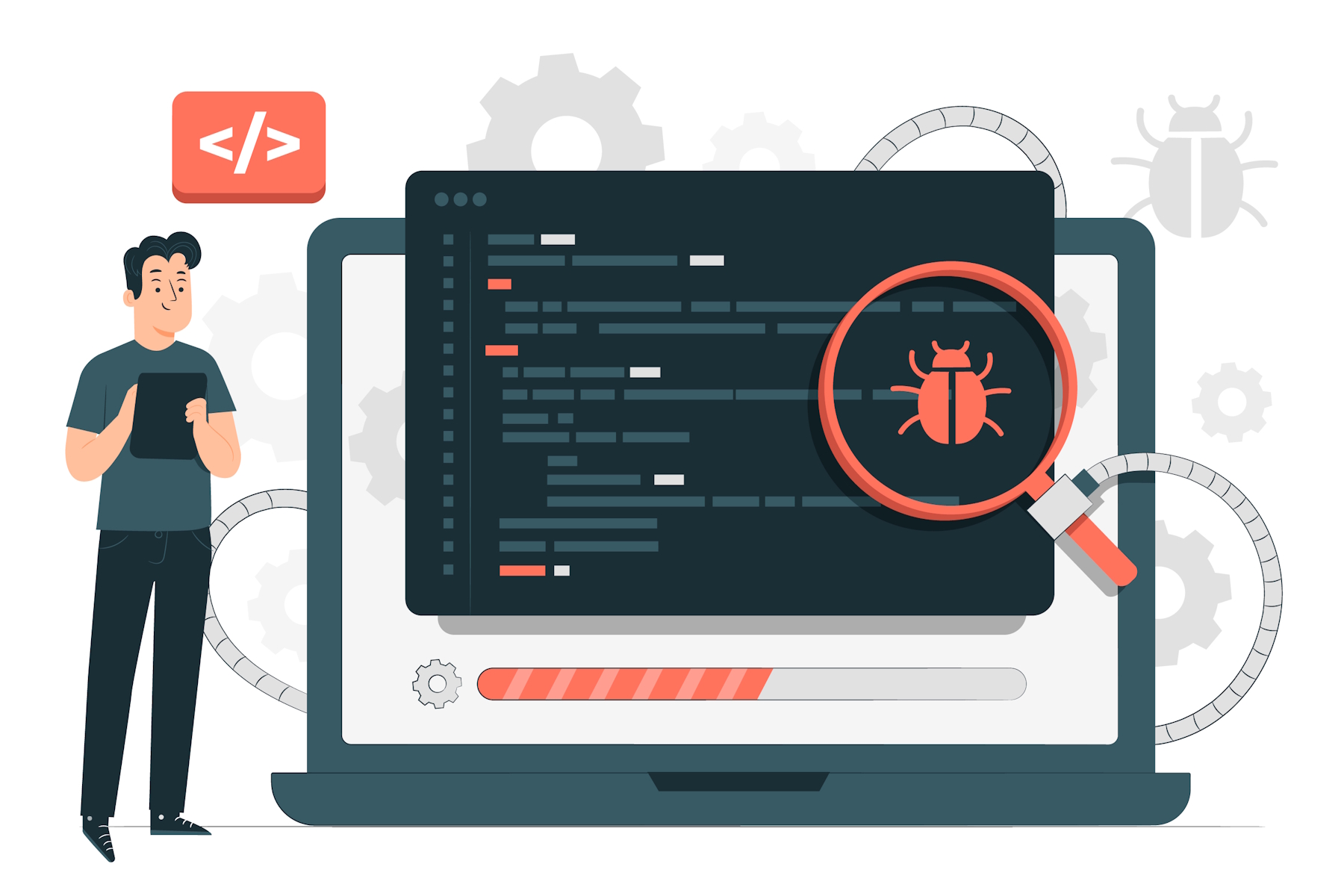
2. Spaghetti Code
Spaghetti code is an unstructured tangle of logic, where control flow and dependencies lack a coherent pattern. Reading or modifying it often feels like unraveling a messy plate of noodles.
Why it’s bad: It leads to brittle systems, frequent regressions, and difficulty onboarding new developers. Understanding cause and effect becomes a guessing game.
How to fix it: Refactor into modular functions, adopt architectural patterns like MVC or MVVM, and consistently apply clean coding principles.
3. Magic Numbers and Strings
These are unexplained constants hardcoded directly into the logic of your application—”magic” because their meaning isn’t immediately clear.
Why it’s bad: Magic values obscure intent, complicate updates, and increase the risk of introducing bugs.
How to fix it: Replace them with named constants or enums, and store configurable values in centralized configuration files or environment variables.
4. Copy-Paste Programming
Copying and pasting code across your project without abstraction is a tempting shortcut—but it creates unnecessary duplication and risk.
Why it’s bad: Bugs propagate easily, and maintaining consistency becomes a nightmare. Refactoring duplicated logic is inefficient and error-prone.
How to fix it: Identify patterns in the duplicated code and extract reusable functions, components, or modules. Follow the DRY (Don’t Repeat Yourself) principle.
5. Premature Optimization
Premature optimization happens when developers focus on improving performance before confirming there’s a problem to solve.
Why it’s bad: It adds unnecessary complexity and may optimize the wrong part of the system, reducing code readability and increasing cognitive load.
How to fix it: Focus on writing clear and correct code first. Use profiling tools to identify performance bottlenecks and optimize based on real data.
6. Lava Flow
Lava Flow refers to legacy code that remains in the system long after its purpose is forgotten. Teams avoid touching it for fear of breaking something.
Why it’s bad: It clutters the codebase and makes onboarding more difficult. It’s also a source of potential bugs and dead weight.
How to fix it: Document legacy code, remove unused functionality during regular cleanups, and rely on version control for historical reference.
7. Shotgun Surgery
This occurs when a single change requires modifying many unrelated files or classes across the system.
Why it’s bad: It makes simple updates laborious and risky, leading to a fragile and bloated architecture.
How to fix it: Group related functionality together and encapsulate it. Aim for high cohesion and low coupling in your system design.
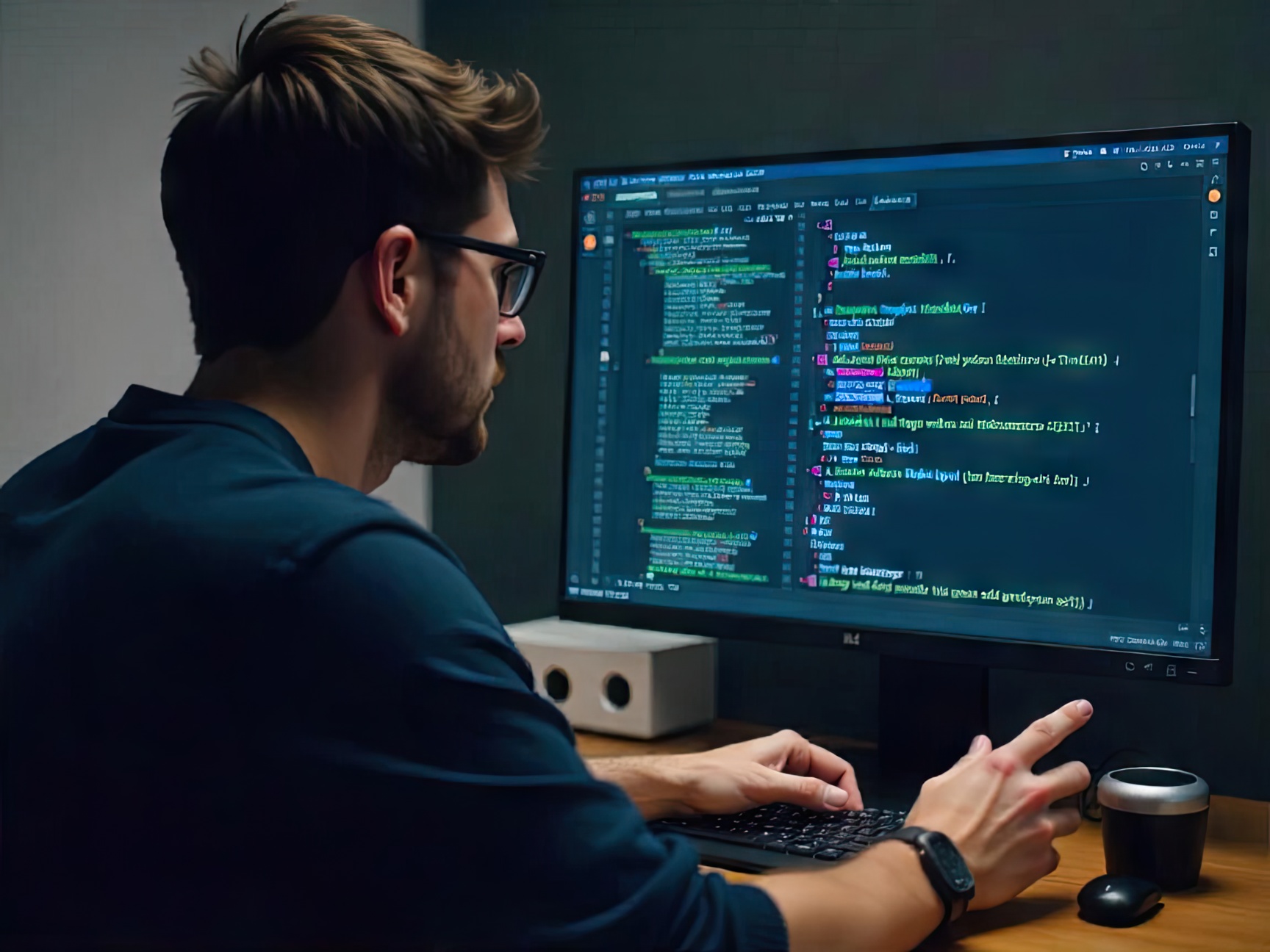
8. Poltergeist Objects
These are short-lived objects that exist only to forward calls or pass data without meaningful behavior or state.
Why it’s bad: They increase architectural noise and provide no clear benefit, making the system harder to understand.
How to fix it: Inline their logic when possible or redesign the structure to eliminate unnecessary indirection.
9. Overengineering
Overengineering is building solutions that are more complex than needed, usually in anticipation of future requirements that may never arrive.
Why it’s bad: It leads to feature creep, increased development time, and harder maintenance.
How to fix it: Apply the YAGNI principle (You Aren’t Gonna Need It). Build minimal, focused functionality and evolve it as real needs emerge.
10. The Golden Hammer
This anti-pattern arises when developers try to apply a single familiar solution or technology to every problem—regardless of suitability.
Why it’s bad: It stifles innovation and often results in mismatched or inefficient implementations.
How to fix it: Stay open to learning new patterns, tools, and paradigms. Let the problem shape the solution—not the other way around.
Conclusion
Anti-patterns are not merely the result of bad code—they’re the accumulation of unchecked habits, rushed decisions, and evolving systems. Recognizing these problematic patterns is the first step toward more reliable and maintainable software.
By avoiding these common traps, you can streamline your development process, reduce technical debt, and foster a more collaborative and confident engineering culture. Encourage code reviews, adopt consistent standards, and maintain a mindset of continuous improvement.
🚫 Final Takeaway: Programming anti-patterns are avoidable. With vigilance, discipline, and curiosity, you can write better code and build more resilient systems.
Your future self—and your teammates—will thank you.