In the ever-evolving world of software development and systems engineering, a deep understanding of how modern operating systems handle multitasking and concurrency is essential. Two core concepts at the heart of this capability are threads and processes. While both enable parallel execution of tasks, they differ significantly in structure, behavior, performance, and use cases.
Whether you’re building a desktop application, a mobile app, a backend service, or a distributed system, making informed choices about threads and processes can dramatically influence your application’s performance, responsiveness, fault tolerance, and overall scalability. The right approach can improve maintainability, enhance user experience, and ensure system reliability.
In this comprehensive guide, we’ll explore what threads and processes are, how they work, their key differences, and when to use each. By understanding these core concepts, developers can make smarter architectural decisions and build more efficient, resilient software systems.
What Is a Process?
A process is an independent instance of a running program. It contains all the resources needed for execution, including its own memory space, program code, open files, system handles, and at least one thread. The operating system treats each process as a self-contained unit with strict boundaries from other processes.
Key Characteristics of a Process:
- Maintains its own virtual memory space (heap, stack, code, and data segments)
- Includes system-level resources like file descriptors, open connections, and environment variables
- Operates in isolation from other processes—memory cannot be shared directly
- Requires explicit inter-process communication (IPC) mechanisms such as pipes, sockets, shared memory, or message queues
- Crash containment: failure in one process generally does not affect others
Practical Examples:
- A web browser where each tab is a separate process to prevent one crashing tab from impacting the entire browser
- An IDE spawning subprocesses for compilation or linting tasks
- A containerized cloud application running user jobs in isolated environments
Processes are excellent for applications requiring fault isolation, security, or independent execution.
What Is a Thread?
A thread is the smallest unit of execution within a process. While a process can have a single thread, most modern applications use multiple threads to run tasks concurrently. Threads in the same process share memory and system resources, but each has its own stack and instruction pointer.
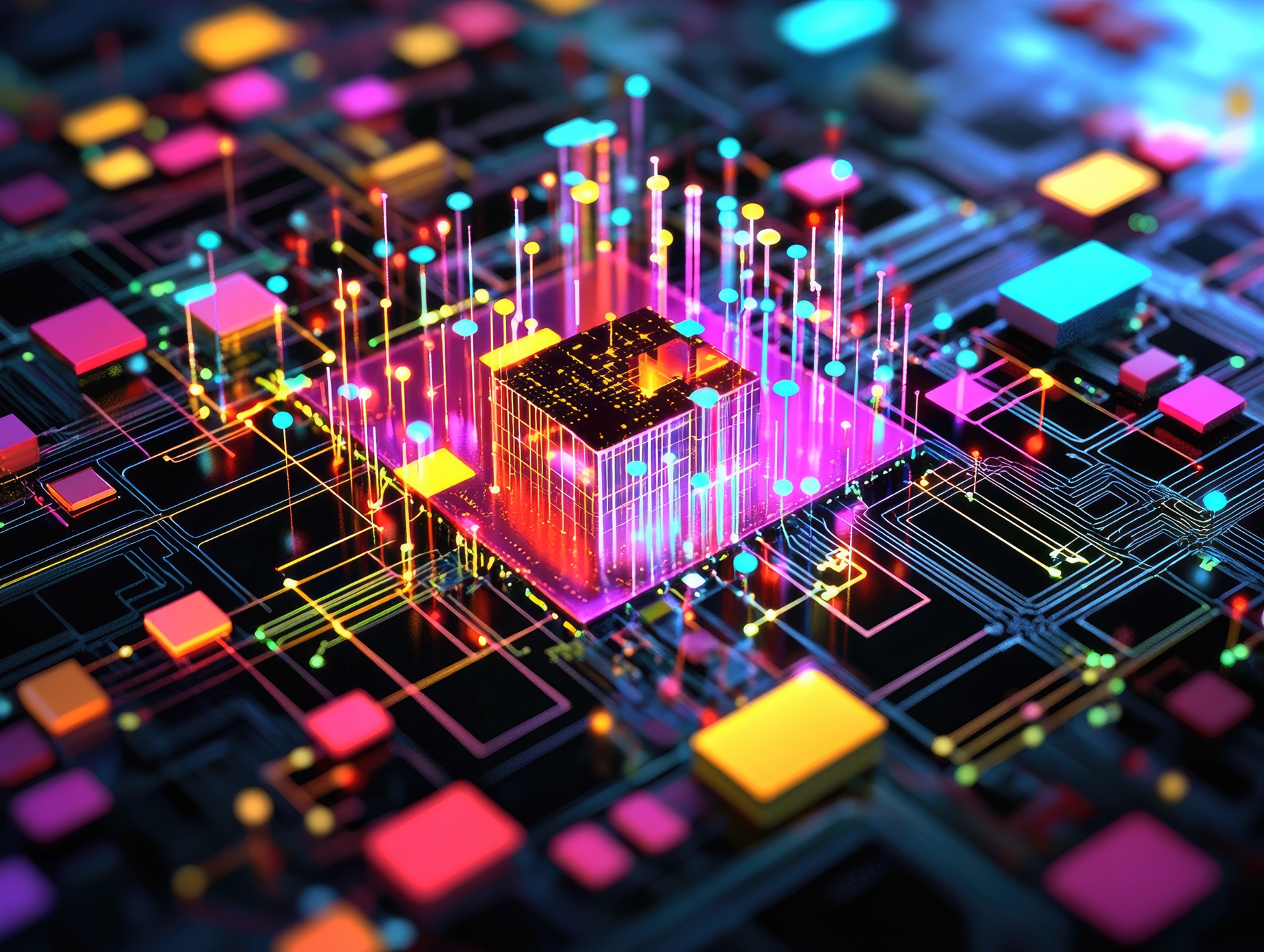
Key Characteristics of a Thread:
- Shares heap memory and system resources (e.g., open files, network connections) with sibling threads
- Has its own stack, registers, and program counter
- Creation and destruction are typically faster than processes
- Allows for direct communication between threads via shared memory
- A fault in one thread (such as a segmentation fault) can bring down the entire process
Practical Examples:
- A text editor using separate threads for autosave, spell check, and UI updates
- A multiplayer game using distinct threads for rendering, physics, and input
- A server that handles each client request with a separate thread to enable concurrent responses
Threads are ideal for applications that require fine-grained concurrency, shared state, and responsiveness.
Key Differences Between Threads and Processes
Understanding the differences between threads and processes is critical when designing software for performance and reliability:
Feature | Process | Thread |
---|---|---|
Memory Space | Separate | Shared within the same process |
Resource Allocation | Independent | Shared (files, memory, sockets) |
Communication | Requires IPC | Direct via shared memory |
Overhead | Higher | Lower |
Creation Time | Slower | Faster |
Fault Isolation | High — crash does not affect others | Low — crash can affect entire process |
Scalability | Good for distributed systems | Good for concurrent tasks within a system |
Debugging Complexity | Easier | More complex due to shared state |
Use Cases | Isolated apps or services | Concurrent tasks within one application |
When to Use Threads vs. Processes
Choosing between threads and processes depends on your application’s needs. Each offers distinct benefits depending on the use case.
Use Threads When:
- Multiple tasks need to share data or memory efficiently
- Low-latency communication is required between components
- You’re building a responsive user interface or real-time system
- The application is I/O-bound and needs to perform concurrent operations (e.g., file and network I/O)
Examples:
- A mobile app downloading content while remaining responsive to user input
- A web server using a thread pool to handle multiple client requests simultaneously
- A streaming service processing video/audio data in real time
Use Processes When:
- Tasks must be isolated for reliability, security, or resource separation
- You need to execute external programs or multi-language code
- You’re building a distributed system where components run independently
- You want to scale horizontally across multiple cores or machines
Examples:
- A microservices-based architecture with services running in separate containers
- A security-focused system sandboxing plugins or user scripts
- A toolchain launching subprocesses for compilation or rendering
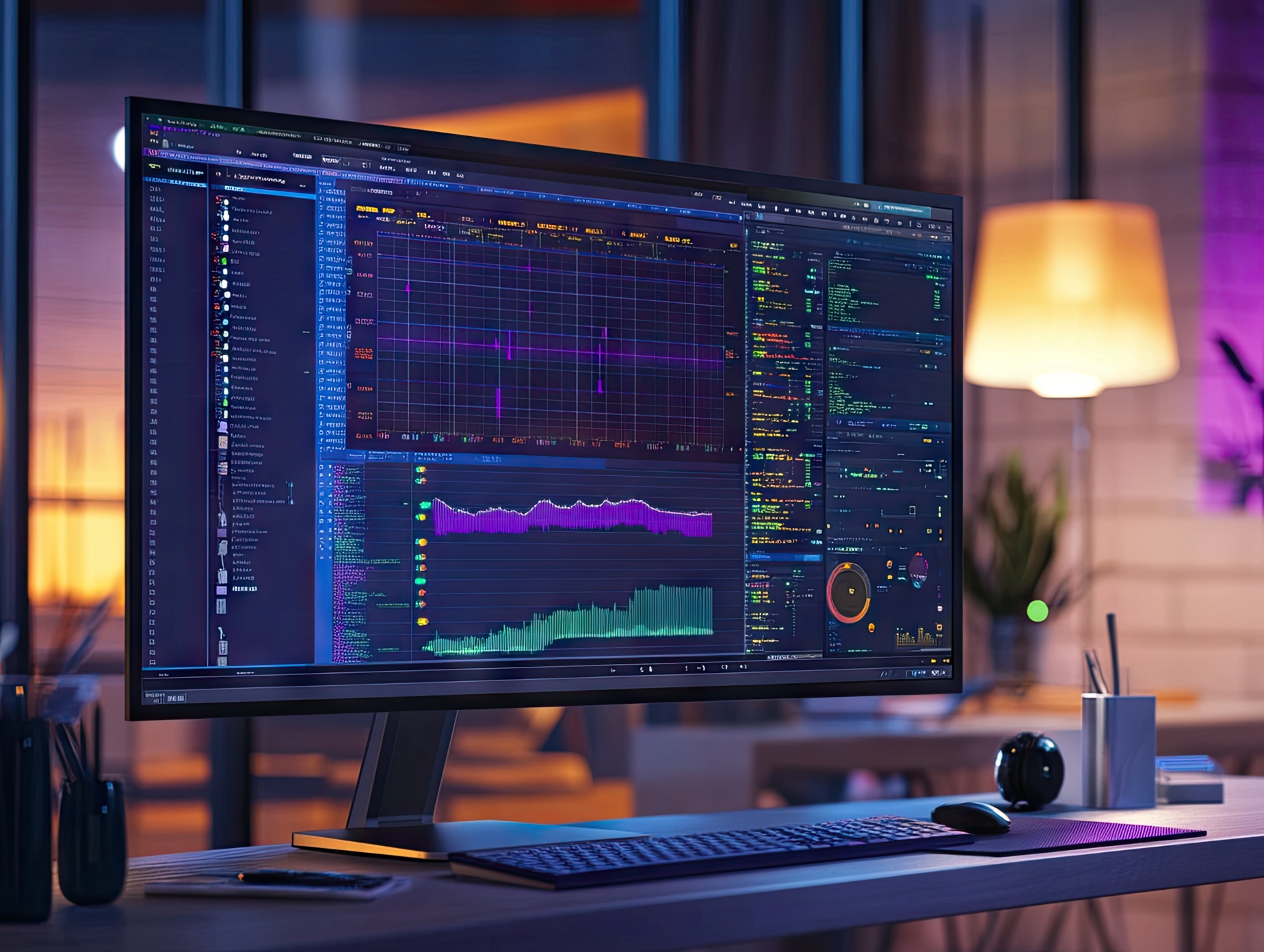
Performance and Scalability Considerations
When optimizing for performance and scalability, the choice between threads and processes plays a significant role.
Performance:
- Threads have lower overhead due to shared memory and faster context switching
- Processes consume more memory and CPU but offer better containment and parallelism on multi-core systems
Scalability:
- Threads excel within a single machine, especially for I/O-bound workloads
- Processes can be distributed across machines or containers, offering horizontal scalability
Complexity:
- Threads require careful handling of synchronization (e.g., locks, semaphores) to avoid race conditions
- Processes simplify isolation but introduce overhead for communication and data sharing
Many high-performance systems combine both paradigms—using processes for modularity and threads for internal concurrency.
Security and Fault Tolerance
Security and fault isolation are key considerations in modern application design:
- Threads share memory, increasing risk if one thread corrupts shared state or encounters a fault
- Processes offer stronger isolation, making them safer for running untrusted code or high-risk tasks
Operating systems use process isolation to enforce security boundaries, which is why containers and virtual machines rely heavily on process-based models.
Conclusion
Threads and processes are foundational concepts in concurrent programming. Choosing between them involves balancing performance, resource sharing, fault isolation, and system complexity.
Threads are excellent for achieving concurrency within an application that benefits from shared memory and efficient task switching. Processes shine when you need isolated execution, better fault containment, and greater scalability across systems.
By mastering the distinctions between these two models, developers can design and implement systems that are fast, robust, and scalable.
🚀 Challenge yourself: Try building a sample application that implements both multithreading and multiprocessing. Benchmark performance, observe behavior under load, and compare how each model handles faults. It’s a hands-on way to gain deep insights into these essential building blocks of modern computing.