Python is celebrated for its simplicity, readability, and versatility, making it a popular choice for both beginners and experienced developers. However, even in a language as user-friendly as Python, errors and bugs are inevitable. Understanding the most common Python errors and how to fix them is essential for writing robust and error-free code. In this post, we’ll explore ten common Python errors, explain why they occur, and provide solutions to fix them.
1. SyntaxError: Invalid Syntax
What It Is:
A SyntaxError
occurs when Python encounters a line of code that does not conform to the correct syntax of the language. This can happen for various reasons, such as missing colons, parentheses, or incorrect indentation.
Example:
if x > 10 print("x is greater than 10")
Why It Happens:
In the example above, the colon :
is missing after the if
statement.
How to Fix It:
Always ensure that your code follows the correct Python syntax. For the above example, you should add the missing colon:
if x > 10: print("x is greater than 10")
Tip:
Use an Integrated Development Environment (IDE) or a code editor with syntax highlighting and linting features, which can help you spot syntax errors more easily.
2. IndentationError: Unexpected Indent
What It Is:
Python relies heavily on indentation to define the structure and flow of the code. An IndentationError
occurs when the code is not indented correctly.
Example:
def my_function():print("Hello, World!")
Why It Happens:
In Python, code blocks should be indented consistently. The print
statement should be indented to indicate that it is part of the my_function()
block.
How to Fix It:
Indent the print
statement correctly:
def my_function(): print("Hello, World!")
Tip:
Use spaces or tabs consistently for indentation, but avoid mixing both. Most Python developers use four spaces per indentation level.
3. NameError: Name ‘x’ is Not Defined
What It Is:
A NameError
occurs when you try to use a variable or function that has not been defined.
Example:
print(x)
Why It Happens:
The variable x
has not been assigned a value before it is used in the print
statement.
How to Fix It:
Ensure that the variable is defined before you use it:
x = 10print(x)
Tip:
Double-check the spelling of variable names to ensure consistency throughout your code.
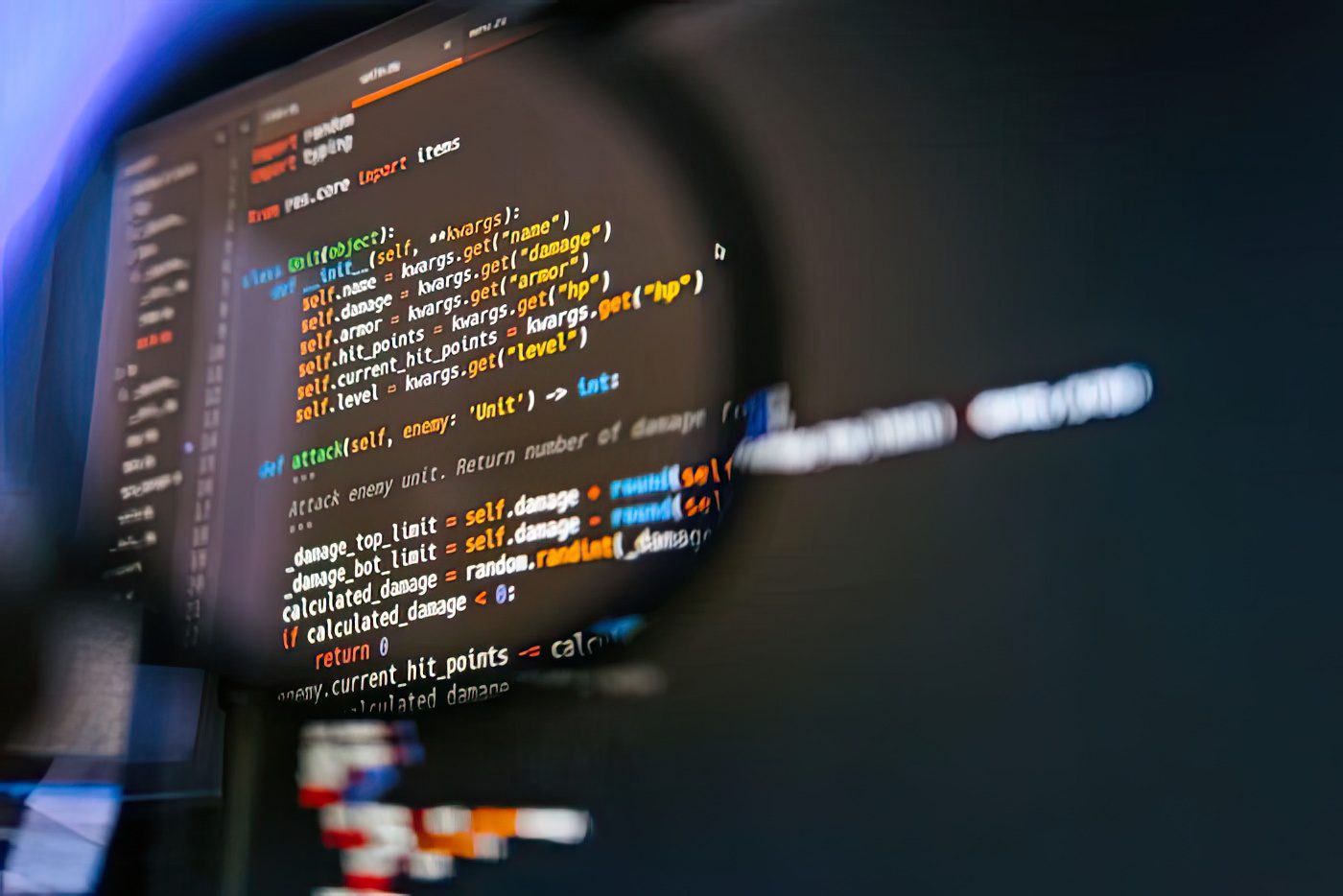
4. TypeError: Unsupported Operand Type(s)
What It Is:
A TypeError
occurs when an operation or function is applied to an object of an inappropriate type.
Example:
result = '5' + 5
Why It Happens:
In the example above, Python cannot add a string ('5'
) and an integer (5
).
How to Fix It:
Convert the string to an integer or the integer to a string before performing the operation:
result = int('5') + 5 # orresult = '5' + str(5)
Tip:
Be mindful of data types and use type conversion functions like int()
, str()
, and float()
as needed.
5. IndexError: List Index Out of Range
What It Is:
An IndexError
occurs when you try to access an index that is out of the range of a list.
Example:
my_list = [1, 2, 3]print(my_list[3])
Why It Happens:
Lists in Python are zero-indexed, meaning the first element is at index 0
. In this example, my_list[3]
is trying to access the fourth element, which doesn’t exist.
How to Fix It:
Ensure the index is within the valid range of the list:
print(my_list[2]) # Accesses the third element
Tip:
Use the len()
function to check the length of the list before accessing an index.
6. ValueError: Invalid Literal for int() with Base 10
What It Is:
A ValueError
occurs when a function receives an argument of the correct type but an inappropriate value.
Example:
number = int("abc")
Why It Happens:
The int()
function expects a string that can be converted to an integer, but "abc"
cannot be converted.
How to Fix It:
Ensure the string contains only digits before converting it:
number = int("123")
Tip:
Use try...except
blocks to handle potential ValueError
exceptions, especially when converting user input.
7. AttributeError: ‘module’ object has no attribute
What It Is:
An AttributeError
occurs when you try to access an attribute or method that doesn’t exist for a given object.
Example:
import mathprint(math.square(4))
Why It Happens:
The math
module does not have a method named square()
. The correct method is sqrt()
.
How to Fix It:
Use the correct attribute or method name:
print(math.sqrt(4))
Tip:
Use Python’s dir()
function to list all attributes and methods available for an object.
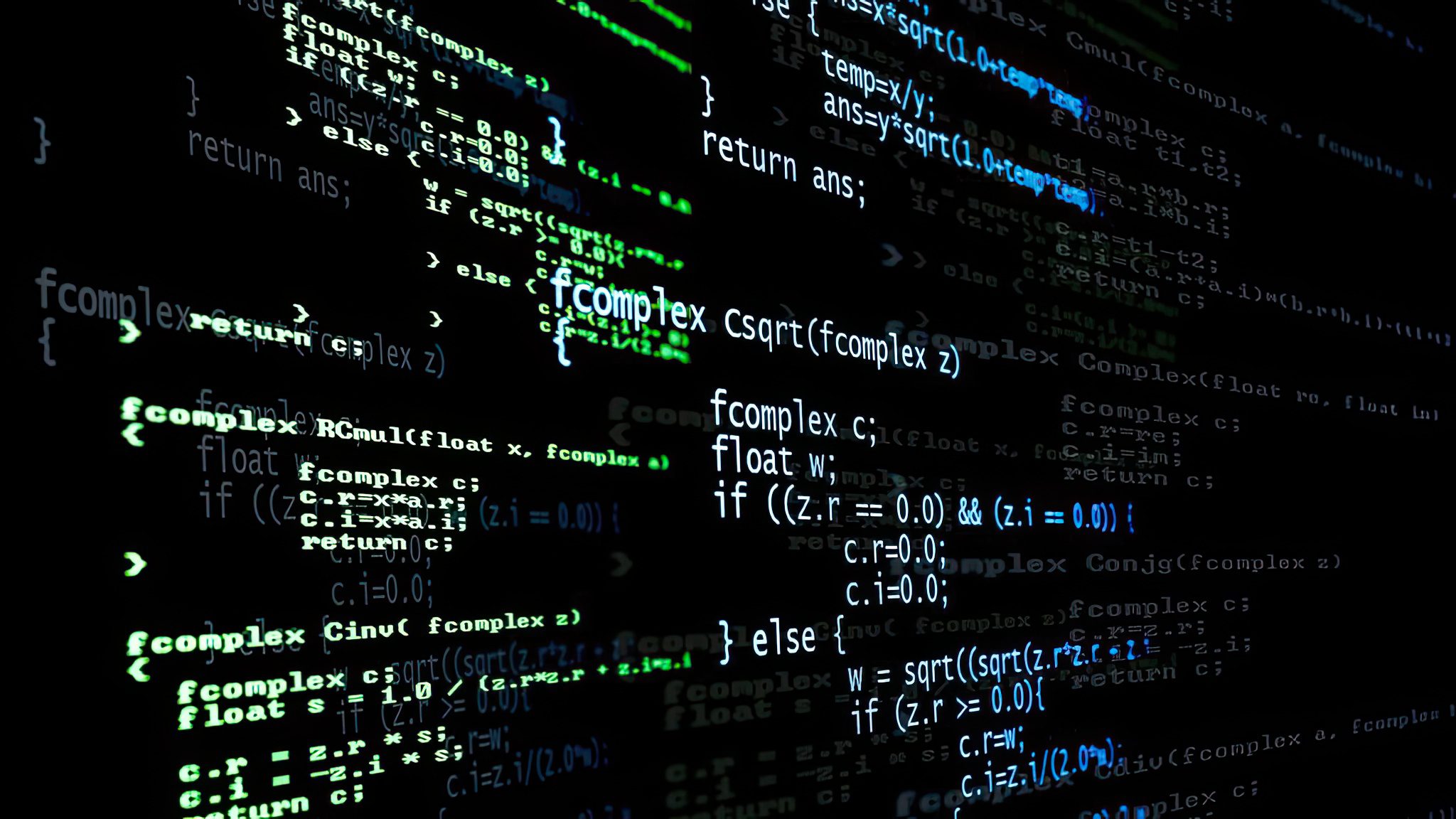
8. KeyError: ‘key’
What It Is:
A KeyError
occurs when you try to access a dictionary key that doesn’t exist.
Example:
my_dict = {"name": "Alice", "age": 25}print(my_dict["gender"])
Why It Happens:
The key "gender"
does not exist in my_dict
.
How to Fix It:
Check if the key exists before accessing it:
if "gender" in my_dict: print(my_dict["gender"])else: print("Key not found")
Tip:
You can also use the get()
method, which returns None
(or a default value) if the key is not found:
print(my_dict.get("gender", "Key not found"))
9. ImportError: No Module Named ‘module’
What It Is:
An ImportError
occurs when Python cannot find the module you are trying to import.
Example:
import non_existent_module
Why It Happens:
The module you are trying to import doesn’t exist or is not installed in your Python environment.
How to Fix It:
Ensure the module is installed and correctly spelled:
pip install module_name
Tip:
Use virtual environments to manage dependencies and ensure all required modules are installed.
10. ZeroDivisionError: Division by Zero
What It Is:
A ZeroDivisionError
occurs when you try to divide a number by zero, which is mathematically undefined.
Example:
result = 10 / 0
Why It Happens:
Dividing by zero is not allowed in mathematics, and Python throws an error when you attempt to do so.
How to Fix It:
Check the denominator before performing the division:
denominator = 0if denominator != 0: result = 10 / denominatorelse: print("Cannot divide by zero")
Tip:
Always validate input values to prevent such errors, especially when dealing with user input or calculations.
Conclusion
Python errors can be frustrating, but they are also opportunities to learn and improve your coding skills. By understanding these common errors and knowing how to fix them, you’ll become more proficient in Python and better equipped to handle any coding challenges that come your way. Always test your code thoroughly, use debugging tools, and don’t hesitate to consult the Python documentation or community when you encounter issues.
This post will guide you through understanding and fixing common Python errors, ensuring you can write more reliable and efficient code.