In the realm of mobile development, two programming languages stand out for their modern features, strong community support, and official backing from major tech companies: Kotlin and Swift. Kotlin, developed by JetBrains, is the preferred language for Android development, while Swift, developed by Apple, is the go-to language for iOS development. But which language is better for mobile development? This comprehensive guide compares Kotlin and Swift across various aspects to help you make an informed decision.
Background and History
Kotlin
Kotlin is a statically typed programming language that runs on the Java Virtual Machine (JVM). It was developed by JetBrains, the company behind IntelliJ IDEA, and officially released in 2011. In 2017, Google announced Kotlin as the preferred language for Android app development, which significantly boosted its popularity.
Key Features of Kotlin:
- Interoperability with Java: Kotlin is fully interoperable with Java, allowing developers to use existing Java libraries and frameworks.
- Concise Syntax: Reduces boilerplate code and enhances readability.
- Null Safety: Built-in null safety features to prevent null pointer exceptions.
- Coroutines: Simplifies asynchronous programming.
Swift
Swift is a powerful and intuitive programming language developed by Apple for iOS, macOS, watchOS, and tvOS app development. It was introduced in 2014 as a replacement for Objective-C, aiming to provide a modern language that is easier to learn and use.
Key Features of Swift:
- Performance: Compiles to native code, offering high performance for applications.
- Safety: Strong type inference and memory management features to prevent errors.
- Modern Syntax: Combines the power and performance of compiled languages with the simplicity and expressiveness of scripting languages.
- Interoperability with Objective-C: Allows the use of existing Objective-C code and libraries.
Language Syntax and Features
Syntax Comparison
Both Kotlin and Swift are designed to be concise, expressive, and easy to read. Here is a side-by-side comparison of their syntax:
Kotlin Example:
fun main() { val greeting = "Hello, Kotlin!" println(greeting) }
Swift Example:
let greeting = "Hello, Swift!" print(greeting)
Observations:
- Both languages use modern, concise syntax.
- Variable declaration in Kotlin uses
val
for immutable variables andvar
for mutable variables, while Swift useslet
for immutable andvar
for mutable variables. - Both languages support type inference, reducing the need for explicit type declarations.
Null Safety
Kotlin:
Kotlin provides built-in null safety features to eliminate null pointer exceptions. By default, variables cannot be null unless explicitly declared as nullable using the ?
operator.
Example:
var name: String = "Kotlin" var nullableName: String? = null
Swift:
Swift also includes robust null safety features with optional types. Variables can be declared as optional using the ?
operator, and unwrapped safely using optional binding.
Example:
var name: String = "Swift" var nullableName: String? = nil if let unwrappedName = nullableName { print(unwrappedName) } else { print("Name is nil") }
Comparison:
- Both languages provide strong null safety features, reducing runtime errors related to null values.
- Kotlin’s null safety is built directly into the type system, while Swift uses optional types to handle null values.
Tooling and IDE Support
Kotlin
Kotlin is supported by a variety of integrated development environments (IDEs), with IntelliJ IDEA and Android Studio being the most popular. These IDEs offer robust features for Kotlin development, including:
- Code Completion: Intelligent code completion and suggestions.
- Refactoring Tools: Advanced refactoring tools to improve code quality.
- Debugging: Comprehensive debugging tools for efficient troubleshooting.
- Kotlin-Specific Plugins: Plugins that enhance the development experience with Kotlin-specific features.
Swift
Swift development is primarily supported by Xcode, Apple’s official IDE for macOS and iOS app development. Xcode provides a powerful set of tools for Swift development, including:
- Code Completion: Context-aware code completion and suggestions.
- Interface Builder: Visual tool for designing user interfaces.
- Debugging: Advanced debugging tools with real-time feedback.
- Playgrounds: Interactive environment for experimenting with Swift code.
Comparison:
- Both Kotlin and Swift benefit from strong IDE support, with Android Studio and IntelliJ IDEA for Kotlin, and Xcode for Swift.
- Each IDE offers a comprehensive set of tools tailored to their respective languages, enhancing the development experience.
Performance and Efficiency
Kotlin
Kotlin’s performance is on par with Java, as it runs on the JVM. It benefits from JVM optimizations and can leverage existing Java libraries and frameworks. Additionally, Kotlin’s coroutines provide an efficient way to handle asynchronous programming, reducing the complexity and improving performance.
Swift
Swift is designed for high performance, compiling to native code for iOS and macOS platforms. It offers various optimizations, such as ARC (Automatic Reference Counting) for efficient memory management. Swift’s performance is comparable to C and C++, making it suitable for performance-critical applications.
Comparison:
- Kotlin and Swift both offer high performance, with Kotlin leveraging JVM optimizations and Swift compiling to native code.
- Swift’s performance is particularly notable for iOS and macOS development, where it can achieve near-native performance levels.
Ecosystem and Community Support
Kotlin
Kotlin has a rapidly growing ecosystem, driven by its official support from Google for Android development. The Kotlin community is vibrant, with extensive documentation, tutorials, and a wide range of libraries and frameworks.
Key Resources:
- Kotlin Documentation: Comprehensive documentation provided by JetBrains.
- Kotlin Slack Community: An active community for discussing Kotlin development.
- Kotlin Libraries: A wide range of libraries for various purposes, from UI development to networking.
Swift
Swift also boasts a strong ecosystem, supported by Apple. The Swift community is active, with a wealth of resources, including official documentation, community forums, and numerous open-source libraries.
Key Resources:
- Swift Documentation: Official documentation provided by Apple.
- Swift Forums: An active community for discussing Swift development.
- Swift Libraries: A rich collection of open-source libraries for various functionalities.
Comparison:
- Both Kotlin and Swift have strong community support and rich ecosystems, providing ample resources for developers.
- The choice between Kotlin and Swift often depends on the target platform (Android or iOS) and the specific requirements of the project.
Use Cases and Industry Adoption
Kotlin
Kotlin is predominantly used for Android app development, but its interoperability with Java makes it suitable for server-side development as well. Many major companies have adopted Kotlin for their Android applications, including:
- Pinterest: Uses Kotlin for its Android app.
- Trello: Migrated to Kotlin for Android development.
- Square: Implements Kotlin in its Android SDKs.
Swift
Swift is the primary language for iOS, macOS, watchOS, and tvOS development. Its performance and modern features have led many companies to adopt Swift for their applications, including:
- Airbnb: Uses Swift for its iOS app.
- Lyft: Migrated to Swift for iOS development.
- LinkedIn: Implements Swift in its iOS applications.
Comparison:
- Kotlin is widely adopted for Android development, while Swift is the preferred language for iOS development.
- Both languages have seen significant industry adoption, with many major companies using them for their mobile applications.
Learning Curve
Kotlin
Kotlin’s syntax is designed to be easy to learn, especially for developers with a background in Java. The language’s modern features, such as null safety and concise syntax, make it appealing to new developers. The availability of extensive documentation and tutorials further eases the learning process.
Swift
Swift is designed to be intuitive and easy to learn, even for beginners. Its modern syntax and strong typing system help prevent common programming errors. Apple provides a wealth of learning resources, including the interactive Swift Playgrounds app, which is particularly helpful for new developers.
Comparison:
- Both Kotlin and Swift are designed to be easy to learn and use.
- Developers with a background in Java may find Kotlin easier to pick up, while those familiar with Apple’s ecosystem may prefer Swift.
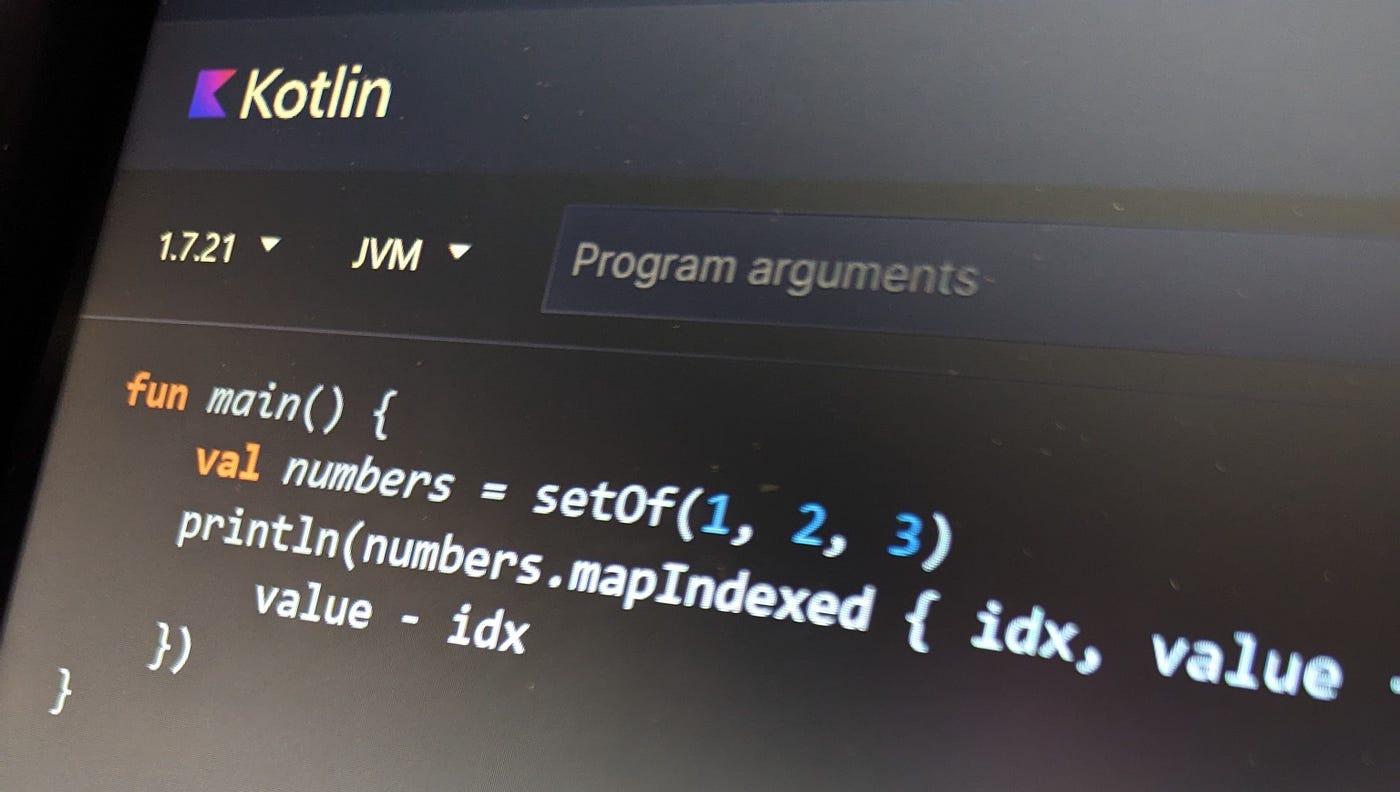
Integration with Development Tools
Kotlin
Kotlin integrates seamlessly with existing Java development tools, enhancing the development experience for Android developers. Key development tools include:
- Android Studio: The official IDE for Android development, which provides excellent support for Kotlin.
- IntelliJ IDEA: A powerful IDE from JetBrains, the creators of Kotlin, offering advanced features for Kotlin development.
- Gradle: A build automation tool that supports Kotlin scripting for project configuration.
Swift
Swift integrates closely with Apple’s development ecosystem, providing a smooth development experience for iOS and macOS developers. Key development tools include:
- Xcode: The official IDE for Apple platform development, offering comprehensive support for Swift.
- Swift Playgrounds: An interactive environment for learning and experimenting with Swift code.
- CocoaPods: A dependency manager for Swift and Objective-C Cocoa projects, facilitating the integration of third-party libraries.
Comparison:
- Kotlin integrates well with Java development tools, making it an excellent choice for Android development.
- Swift is tightly integrated with Apple’s development tools, providing a seamless experience for iOS and macOS development.
Community and Support
Kotlin
Kotlin benefits from a strong and active community, with numerous resources available for learning and support. Key community resources include:
- Kotlin Slack: An active community where developers can ask questions and share knowledge.
- Stack Overflow: A popular platform for finding answers to Kotlin-related questions.
- KotlinConf: An annual conference dedicated to Kotlin development, featuring talks and workshops from industry experts.
Swift
Swift also boasts a vibrant community, supported by Apple and the broader developer ecosystem. Key community resources include:
- Swift Forums: An official platform for discussing Swift development and sharing knowledge.
- Stack Overflow: A valuable resource for finding solutions to Swift-related questions.
- WWDC (Apple Worldwide Developers Conference): An annual event where Apple announces new technologies and provides sessions on Swift and iOS development.
Comparison:
- Both Kotlin and Swift have strong, supportive communities that provide valuable resources for developers.
- Community support and resources can significantly enhance the learning and development experience for both languages.
Real-World Examples
Kotlin in Use
Pinterest: Pinterest uses Kotlin for its Android app development, leveraging Kotlin’s concise syntax and interoperability with Java to improve code quality and developer productivity.
Trello: Trello migrated to Kotlin to benefit from its modern features and improved code maintainability. The transition to Kotlin has helped Trello’s development team write more efficient and reliable code.
Swift in Use
Airbnb: Airbnb uses Swift for its iOS app development, taking advantage of Swift’s performance and modern syntax to deliver a smooth and responsive user experience.
Lyft: Lyft migrated to Swift to enhance its iOS app’s performance and maintainability. Swift’s safety features have helped Lyft reduce bugs and improve app stability.
Future Prospects
Kotlin
Kotlin’s future looks promising, particularly with its strong backing from Google and its increasing adoption in the Android development community. Additionally, Kotlin is expanding beyond mobile development into areas like server-side development, web development, and multiplatform projects with Kotlin/Native and Kotlin/JS.
Swift
Swift continues to evolve under Apple’s stewardship, with regular updates and enhancements. Its adoption is likely to grow as Apple continues to innovate in the iOS and macOS ecosystems. Swift is also expanding its reach with server-side development through Swift on the server and initiatives like Swift for TensorFlow.
Comparison:
- Both Kotlin and Swift are evolving rapidly, with growing ecosystems and expanding use cases.
- Developers should consider future trends and the long-term support of each language when choosing between Kotlin and Swift.
Conclusion
Choosing between Kotlin and Swift for mobile development ultimately depends on your target platform and specific project requirements. Kotlin is an excellent choice for Android development, offering modern features, strong interoperability with Java, and robust IDE support. Swift, on the other hand, is the preferred language for iOS and macOS development, providing high performance, safety, and seamless integration with Apple’s development tools.
Both languages have strong communities, rich ecosystems, and promising futures, making them valuable skills for any mobile developer. By understanding the strengths and nuances of each language, developers can make informed decisions that best align with their goals and project needs.
Useful Links
- Kotlin Documentation
- Why Kotlin for Android Development?
- IntelliJ IDEA for Kotlin Development
- Xcode for Swift Development
- Swift Programming Language
- Kotlin Multiplatform
- Swift.org
- Pinterest Engineering
- Airbnb Engineering
By comparing Kotlin and Swift across various aspects, including syntax, tooling, performance, community support, and industry adoption, developers can better understand which language suits their mobile development needs. Both languages offer unique advantages and are powerful tools in the hands of skilled developers.
Join the Cosmic Meta Community!
Stay ahead of the tech curve with expert insights, cutting-edge trends, and practical tips delivered straight to your inbox. Subscribe now and unlock the power of the digital age!